Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial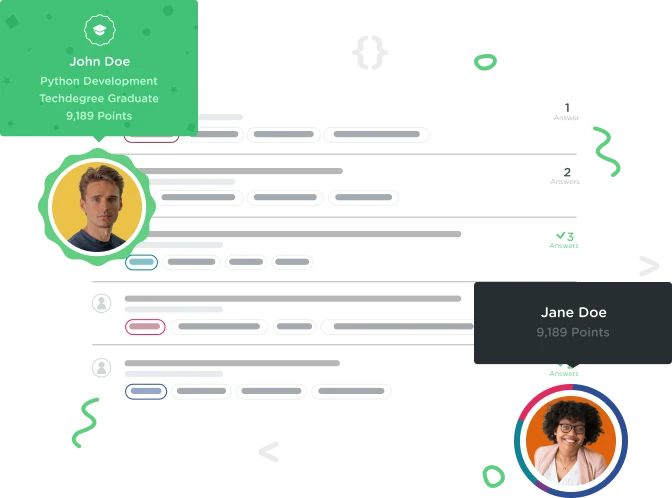

Iain Simmons
Treehouse Moderator 32,305 PointsFormatting date strings
Guil Hernandez, one thing that I did differently is formatting the date strings. Firstly, because I'm from Australia and I don't like the US date format, and secondly, because using the getMonth()
etc methods are a drag!
Here's mine:
let now = new Date();
now = `${now.toLocaleDateString('en-AU')} ${now.toLocaleTimeString('en-AU')}`;
Here are the docs for anyone who is interested in these Date methods:
You can also specify some additional options to those methods to get things like the name of the month in a specific locale, etc.
Should be handy for any other international students here at Treehouse! :)
5 Answers

Evan Goodwin
Courses Plus Student 22,260 PointsI have two issues.
1) You can't remove players anymore because the link to the red X is in the 'player-name' div. Anywhere you click in the 'player-name' div triggers selectPlayer and shows the PlayerDetail.
2) I tried adding a date/time stamp for the 'updated' and 'created' fields. If you keep the date/time generator outside of the Player function, the time stays stuck at the time the page first loads and doesn't change when you update a score. I moved the date/stamp (following Mr. Simmons above) inside the ADD_PLAYER case and UPDATE_PLAYER_SCORE cases as so:
case PlayerActionTypes.ADD_PLAYER: {
var date = new Date();
var fullDate = date.toLocaleDateString();
var fullTime = date.toLocaleTimeString();
case PlayerActionTypes.UPDATE_PLAYER_SCORE: { var date = new Date(); var fullDate = date.toLocaleDateString(); var fullTime = date.toLocaleTimeString();
However, this isn't very DRY. How do you generate the date/time stamp in a single place then just call it inside the two cases?

Guil Hernandez
Treehouse TeacherThanks for sharing your solution, Iain Simmons! :)

Dan Avramescu
11,286 PointsI saw the bubbling bug too, and now I see your question :) But event.preventDefault() won't help here. https://teamtreehouse.com/community/i-think-that-here-is-a-small-application-bug

VijayaLakshmi Kalidindi
Courses Plus Student 1,285 Pointscan we use created: Date.now(); For getting the current date?

Iain Simmons
Treehouse Moderator 32,305 PointsNo, Date.now()
will return the number of milliseconds elapsed since 1 January 1970 00:00:00 UTC (the 'UNIX Epoch') as an integer.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date/now
You want to use new Date()
because it creates a new Date
object using its constructor function, and it's that object that contains all the date methods.
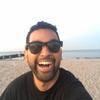
Anthony Albertorio
22,624 PointsI used new Date().toLocaleDateString(). You can pass in your country's code and it will format to it. The method formats to US by default. The example from the MDN docs are as follows:
...
var options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' };
console.log(event.toLocaleDateString('de-DE', options));
// expected output: Donnerstag, 20. Dezember 2012
...
My code looks like:
...
export default function Player(state=initialState, action) {
switch(action.type) {
case PlayerActionTypes.ADD_PLAYER:
const addedPlayer = [ ...state.players, {
name: action.name,
score: 0,
created: new Date().toLocaleDateString(),
updated: new Date().toLocaleDateString()
}];
return {
...state,
players: addedPlayer
};
...
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsBecause the cross/link for deleting players is in the div, the click event will 'bubble' up the DOM tree. You can call
event.preventDefault()
orevent.stopPropogation()
to prevent this (need to haveevent
as a parameter).As for the dates, you can initialize and assign a value to the date variable inside the reducer function, that way it will be set for both the
ADD_PLAYER
andUPDATE_PLAYER_SCORE
actions.