Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial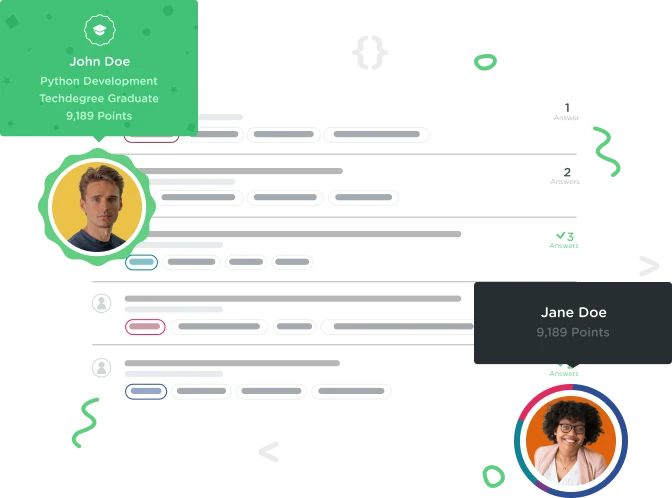
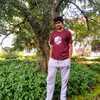
Kailash Seshadri
3,087 PointsFormatting twice in a print() statement
What do I do, if I want to format twice in a print function. For example:
name = input ('What is your name?')
num_tickets = input ('How many tickets would you like?')
print(Thank you for buying {} tickets, {})
#1st {} should be the num_tickets, 2nd {} is the name
I tried this at the start, but it popped up with an error:
print('Thank you for purchasing {} tickets, {}!'.format(num_tickets).format(name))
After some playing around with the code, I found out that this works:
print('Thank you for purchasing {} tickets,'.format(num_tickets), '{}!'.format(name))
I don't know if this is the most efficient way to do it since it takes a while to type out, and want to know if there is a simpler way to approach this.
Thanks!
3 Answers
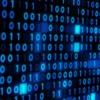
Alexander Davison
65,469 Points.format()
lets you take multiple arguments. Each argument corresponds to one particular {}
.
print("Thank you for buying {} tickets, {}!".format(num_tickets, name))
By default, the first argument of format
corresponds to the first {}
, the second argument to the second {}
, etc. But you can change the order by putting an index number in-between the {}
. Using this is not very common, but for the sake of example, I'll show you:
# The below line prints "Apples and Bananas"
print("{}s and {}s".format("Apple", "Banana"))
# The below line prints "Bananas and Apples"
print("{1}s and {0}s".format("Apple", "Banana"))
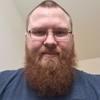
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsAnother thing you can do is to use f-strings which (in my opinion) looks a lot cleaner :-)
name = input ('What is your name?')
num_tickets = input ('How many tickets would you like?')
print(f'Thank you for buying {num_tickets} tickets')
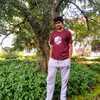
Kailash Seshadri
3,087 PointsF-strings are new to me and that looks great! Thanks!
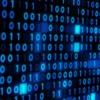
Alexander Davison
65,469 PointsAgreed! f-strings are much nicer.
However, they are only available in Python 3.6
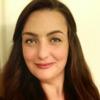
Jennifer Nordell
Treehouse TeacherHi there! Your instincts are correct, and there is a slightly shorter way to do this. You can simply pass the variables in to the format
function separated by a comma. The values will be inserted in order of the {}
found in the string. Take a look:
print('Thank you for purchasing {} tickets, {}!'.format(num_tickets, name))
Hope this helps!
Kailash Seshadri
3,087 PointsKailash Seshadri
3,087 PointsSo using this logic, I should be able to push out strings in any order? For example:
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsYup!
(Try out your code in the Python Shell, it should print out what you expect it to!)
Maxmillan Muchena
2,478 PointsMaxmillan Muchena
2,478 PointsI was wondering the same( actually got stuck and had to changed my code). Thanks for that clear and great response
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsNo problem :)