Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial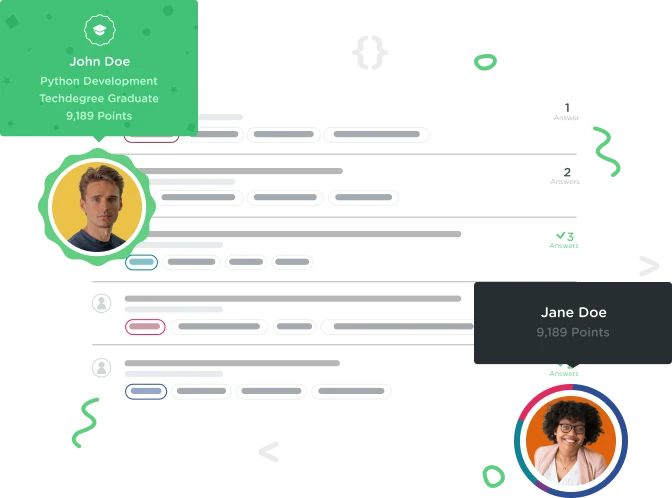

MICHAEL FOWLER
921 PointsFormatting Variables & ints
Two Questions:
- Why do we need to format variables? Example:
# Gather the user's name and assign it to a new variable
name = input("What is your name? ")
# Prompt the user by name and ask how many tickets they would like
num_tickets = input("How many tickets would you like, {}? ".format(name))
My question is, why do I need to format the variable name? Is there reason why I simply cannot type "name" in the second question instead of using the placeholder for name and then the .format(name)?
- Similar questions with ints
Example:
# Prompt the user by name and ask how many tickets they would like
num_tickets = input("How many tickets would you like, {}? ".format(name))
num_tickets = int(num_tickets)
Why do I need to turn the num_tickets into an int? Does Python not read num_tickets as an integer when it initially prompts the user?
Thank you!
[MOD - added ```python formatting -cf]
4 Answers
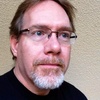
Chris Freeman
Treehouse Moderator 68,423 PointsHi Michael! You may be misinterpreting the purpose of the string method format()
.
In the line:
num_tickets = input("How many tickets would you like, {}? ".format(name))
it is the string "How many tickets would you like, {}" that is getting formatted by inserting the string held in the variable name
into the placeholder {}.
This could be done without using the string format
method. A concatenation version would look like:
num_tickets = input("How many tickets would you like, " + name + "? ")
Using format()
is not required. It is more of a coding style that many feel looks cleaner. Starting with Python3.6, there is also the new f string format:
num_tickets = input(f"How many tickets would you like, {name}? ")
Post back if you have further questions. Good luck!!

MICHAEL FOWLER
921 PointsHi Chris. Thanks you for clearing that up. I do see the difference now between the concatenation version and the other formatted examples and how it is a style preference.
Thanks for taking the time to answer..
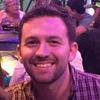
Nick Moy
1,061 PointsHello,
I was wondering why we have to reassign num_tickets = int(num_tickets)
in order for python to recognize that math is being done here?
Could someone help me better understand this logic?
Thanks!
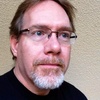
Chris Freeman
Treehouse Moderator 68,423 PointsThe goal is to get an integer value for the number of tickets assigned to the variable num_tickets
based on input from the user. Since user input is received as a string, it will need to be converted using int()
. But why use the form below, you ask?
num_tickets = int(num_tickets)
The above line contains two steps:
- take the object pointed to by
num_tickets
convert to anint
, and return it. - reassign
num_tickets
to point to the object returned by the conversion
Keep in mind that num_tickets
is just a label pointing to some object in memory. As illustrated below, the object id (what itβs pointing to) changes after the new assignment:
>>> num_tickets = '10'
>>> num_tickets
β10β
>>> id(num_tickets)
4584152120
>>> num_tickets = int(num_tickets)
>>> num_tickets
10
>>> id(num_tickets)
4344666944
The following code snippets produce the same results:
num_tickets = input("How many tickets would you like, {}? ".format(name))
num_tickets = int(num_tickets)
str_tickets = input("How many tickets would you like, {}? ".format(name))
num_tickets = int(str_tickets)
num_tickets = int(input("How many tickets would you like, {}? ".format(name)))
Post back if you need more help. Good luck!!!
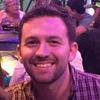
Nick Moy
1,061 PointsThat was very helpful! Thank you, Chris.