Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial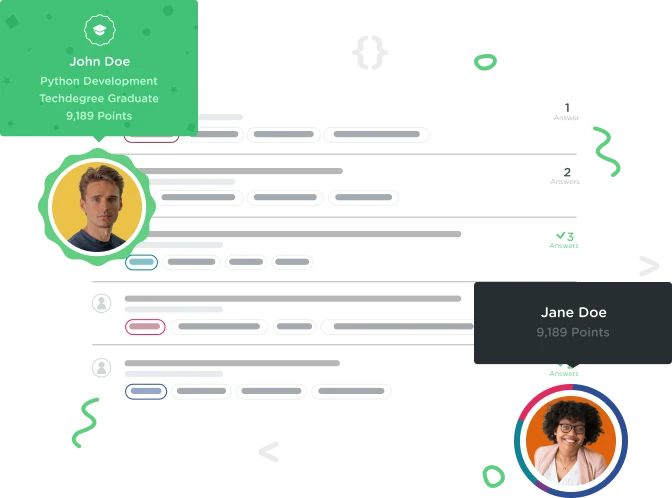

Asaad Khattab
38,121 PointsFortune Cookie Lotto Generator
Hello TreeHouse Community! I'm trying to use Andrew's dice code to generate 6 random number(instead of 1 random number for the die) from 1-49 for my Fortune Cookie Lotto Generator. I tried using a loop but it didn't work. It did work when I added document.write but it took me to another page instead of staying on the same page. I included all the html, css, and javascript code. So our lucky number should be something like this: 33 2 40 12 8 44 I really appreciate your help JAVASCRIPT
function fortuneCookie(range) {
this.range = range;
this.breakCookie = function () {
var randomNumber = Math.floor(Math.random() * this.range) + 1;
return randomNumber;
}
}
var counter = 0;
while ( counter <= 6 ) {
var number = new fortuneCookie(49);
counter += 1;
}
function lotto(number) {
var placeholder = document.getElementById("lottoDisplay");
placeholder.innerHTML = number;
}
/* button */
var button = document.getElementById("fortune-button");
button.onclick = function() {
var total = number.breakCookie();
lotto(total);
};
CSS
body {
background: #384047;
}
.fortune-cookie-h1 {
color: #fff;
}
.program {
display: block;
margin: 200px auto;
padding: 40px;
text-align: center;
width: 80%;
background-color: #fff;
border-radius: 10px;
}
#fortune-button {
display: block;
margin: 0 auto;
padding: 12px 20px;
font-weight: 400;
width: 250px;
border: 3px solid;
font-size: 1em;
color: #fff;
background-color: #384047;
}
#lottoDisplay {
margin: 20px auto;
height: 50px;
width: 250px;
border: 1px solid;
border-radius: 1em;
font-size: 1.5em;
background-color: #fff;
}
#lottoDisplay:after {
color: #4b515d;
}
HTML
<div class="program">
<h1 class"fortune-cookie-h1">Fortune Cookie Lotto Number</h1>
<p id="lottoDisplay">
</p>
<button id="fortune-button">My Number</button>
</div>
The original Javascript code is:
function Dice(sides) {
this.sides = sides;
this.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
}
var dice = new Dice(6);
function printNumber(number) {
var placeholder = document.getElementById("placeholder");
placeholder.innerHTML = number;
}
var button = document.getElementById("button");
button.onclick = function() {
var result = dice.roll();
printNumber(result);
};
3 Answers
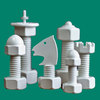
Steven Parker
231,275 PointsYou don't need that current loop at all. But you could do this in the button handler:
button.onclick = function() {
var number = new fortuneCookie(49);
var total = "";
for (var i = 0; i < 6; i++) {
total += number.breakCookie() + " ";
}
lotto(total);
};
BTW, I heard just today on the radio someone WON the lotto by using an actual fortune cookie!
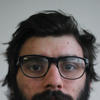
Zack Lee
Courses Plus Student 17,662 PointsI notice that you are trying to create a unique instance of number in a while loop. the problem with this is that each iteration overwrites the previous value.
1) I would replace the while loop with a for loop.
this wont solve the problem, but its cleaner and more compact and will play nicely with the next things you wanna do. which is to place the loop inside the onClick handler. that way it is only fired when the button is clicked. the way its set up now the loop runs as soon as the page loads.
2) i would take what you have in the click handler and place it within your loop, then place that in onClick.
i think this alone will not solve your problem because each iteration will just overwrite whats in the lottoDisplay.
so i would try to push the result of each iteration to an array and then display the array when the loop ends.
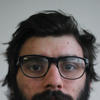
Zack Lee
Courses Plus Student 17,662 Pointslet me know if you need help this code examples. This is just my theory, i haven't put it into syntax.

Asaad Khattab
38,121 PointsThank you Zack!

Antti Lylander
9,686 PointsI have made a lottery simulator, too! Here is how I created the row (random40 is a funtion that makes a random number 1-40). The row is stored in an array and when new random number is created it will be added to the array only if it's index is -1, i.e. there is no same number already.
function createPlayerRow() {
playerRow = [];
do {
let ball = random40();
if ( playerRow.indexOf(ball) === -1 ) {
playerRow.push(ball);
}
} while ( playerRow.length < 7 );
};
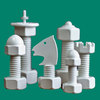
Steven Parker
231,275 PointsGood job!
Now for an extra challenge, can you write a method guaranteed to pick unique numbers each time with no looping?

Asaad Khattab
38,121 PointsNice! Thank you for sharing...

Antti Lylander
9,686 PointsSteven, maybe if i store all 40 possible numbers in an array (like the balls on lottery are in the basket). Then i randomly pick first and add it on the resulting row. Then there are 39 numbers left and I pick the second. Just need to adjust the functions a bit. Like use array lenght as a parameter for random number generator. And so on. This way I guess I don't need a loop. I just need 7 steps and I'll get 7 unique numbers.
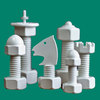
Steven Parker
231,275 PointsExcellent, that's exactly how I would do it.
Actually I have done just that as a developer for a lottery equipment manufacturer.

Antti Lylander
9,686 PointsGreat :)
Here is my lottery simulation in action: http://mimas.kapsi.fi/lotto/
It was my first personal project and I should get back to it now that I have learned a lot more. Most of the functions were written when I had just heard about arrow functions and thought they should be used everywhere. :D
The biggest issue with my simulator is performance. It is too slow on older machines. Probably my methods on creating and checking the rows are not the fastest possible.
I plan to add some options and make the results more informative. Like if you get 7-match on week 20 million it would be fun to know how much you spent on the lottery and how many times you got match 6 or 5.
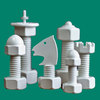
Steven Parker
231,275 PointsVery cool. It might be fun to also show the time in years.
And don't forget to fix the spelling of "lenght" ("length").

Antti Lylander
9,686 PointsThanks!
Yes, time in years would be much more comprehensive. As for spelling, these are impossible to me: length, height, weight. There is no logic in the order of the letters. :D
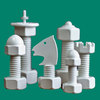
Steven Parker
231,275 PointsIt could be that they sound more alike with a Scandinavian accent.

Antti Lylander
9,686 PointsCan't resist to correct this: Finland is not in Scandinavia actually. ;) A common mistake that many of the Finns also do.
Swedish language is related to English but Finnish is totally something else. Anyhow, now that I tried to pronounce those words, I think I can tell the difference. Thanks!
Asaad Khattab
38,121 PointsAsaad Khattab
38,121 PointsThe code works! I'm very excited. I've been on it for a long time. I don’t recommend anyone using my code on an actual lotto. Haha! Thank you very much Steven.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsTo be a perfect lotto picker you'd still need to make sure all numbers in each set are unique. Once that's done, it would be just as good as a "quick pick"!