Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial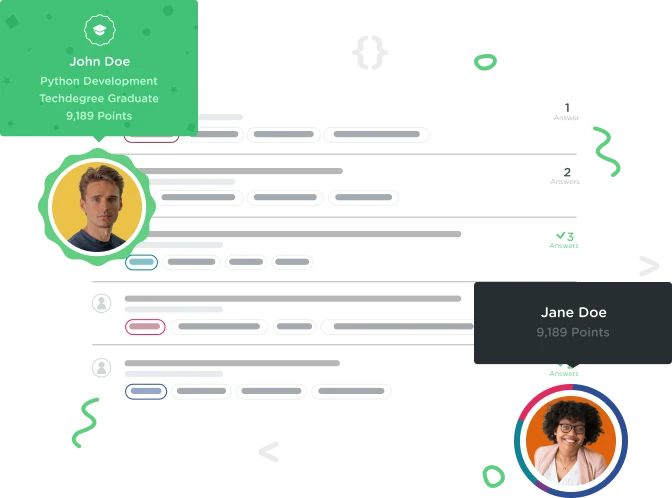

Marc Sanchez
20,039 PointsFound a little bug in the code with changing description and adding to the list.
If a User clicks the 'Change Description List' button, it will replace the description with 'blank': If a User clicks the 'Add Item' button, it will add a blank list item.
I fixed it in my code by adding conditions to both addEventListerner functions.
// Add variables to change description based on user's input
const descriptionP = document.querySelector('p.description');
const descriptionInput = document.querySelector('input.description');
const descriptionButton = document.querySelector('button.description');
// This is the function to change the description based on user's input
descriptionButton.addEventListener('click', () => {
if (descriptionInput.value === '') {
alert("You must enter a change list description");
} else {
descriptionP.textContent = descriptionInput.value + ':';
descriptionP.style.color = 'red';
descriptionInput.value = '';
}
});
// Add variables to add a list item based on user's input
const userInput = document.querySelector('input.addItemInput');
const inputButton = document.querySelector('button.addItemButton');
// This is the function to create a new list item dynamically based on user's input
inputButton.addEventListener('click', () => {
if (userInput.value === '') {
alert("You must enter a change list description");
} else {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = userInput.value;
ul.appendChild(li);
userInput.value = '';
}
});
2 Answers
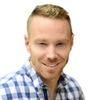
professionalb
Full Stack JavaScript Techdegree Student 5,082 PointsThis is awesome Marc. I would argue that these issues are indeed defects (aka: bugs). Any QA professional would certainly report this issue as a bug (and it would be fixed). Sure, a developer could say, "There was no requirement that stated you could not add an empty item to the list or change the list description to a blank value", but come on, some basic requirements are implicit. In the real world, this would absolutely be a bug regardless of the presence of requirements documentation. This is why we do End-to-End or User Acceptance testing.
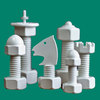
Steven Parker
231,269 PointsI'm not sure if I'd say the original code had a "bug", but your modification of adding input validation is a nice enhancement.

Marc Sanchez
20,039 PointsThank you.
Marc Sanchez
20,039 PointsMarc Sanchez
20,039 PointsI totally agree professionalb. Funny you mentioned QA. I've been a QA eng. for 8 years now and it is something I'd report. Like you said, "This is why we do End-to-End or User Acceptance testing." Ive had my share of debates on whether it's a bug or a requirement issue. As long as it gets fixed it can be called whatever they want to call it. Thanks for your comment.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsMy hesitation to label this a "bug" was due to it being a demonstration project for teaching specific concepts. These projects are often intentionally simplified to keep the focus on the lesson concepts being illustrated.
If you treat it as a piece of functional software (as you would perform user acceptance testing on), there's no question that it's a bug, or at least an incomplete implementation.