Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial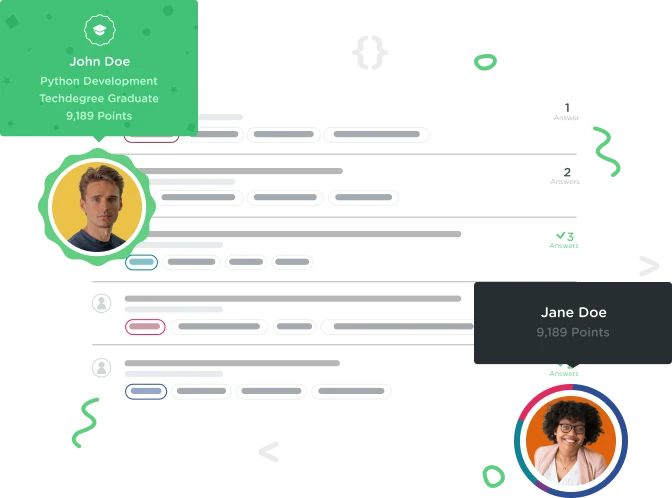

Ganta Hemanth
6,460 PointsFragments in Android
I created a class which is a extends dialog fragment. which is
public class AlertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle("Oops sorry!").setMessage("There was an error. Please try again").setPositiveButton("OK",null);
AlertDialog dialog = builder.create();
return dialog;
}
}
The main activity uses the fragmet in the following code.
private void alertUser() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(),"Error_dialog");
}
How does the type of dialog in main activity match with the type of dialog that is being returned from the AlertDialogFragment??
How does getFragmentManager() work? What does it return??
1 Answer

james white
78,399 PointsHi Ganta,
This is a fairly complex subject,
but I will try to provide a little bit of overview to answer your questions:
How does getFragmentManager() work? What does it return??
First off this question frequently arises:
Should I use getFragmentManager() vs getSupportFragmentManager()?
The answer (briefly) is:
If you are using API >= 14, then use getFragmentManager() and while using Support Package you have to use getSupportFragmentManager()
per this StackOverFlow thread:
http://stackoverflow.com/questions/25306197/using-getfragmentmanager-vs-getsupportfragmentmanager
As for an overview of some of the trickiness in using a DialogFragment here is a two part article:
http://android.codeandmagic.org/why-android-dialogfragment-confuses-me-part1/
http://android.codeandmagic.org/android-dialogfragment-confuses-part-2/
There is a link at the end of part 2 that's very important (concerning avoiding Activity state loss):
http://www.androiddesignpatterns.com/2013/08/fragment-transaction-commit-state-loss.html
After reading through those "overview articles" you should know that the Fragment Manager is not one big monolithic thing.
Instead, when dealing with the Fragment Manager,
it sometimes comes in handy to know a little bit about something called 'Fragment Transactions':
http://developer.android.com/reference/android/app/FragmentTransaction.html
Here's a brief article about that explains a little more (and also talks about the "Backstack" toward the end):
http://www.javacodegeeks.com/2013/06/android-fragment-transaction-fragmentmanager-and-backstack.html
..and I'm sure you've already glanced over the Android Dialogs page:
http://developer.android.com/design/building-blocks/dialogs.html
..as well as the Android Doc 'AlertDialog' page:
http://developer.android.com/reference/android/app/AlertDialog.html
So, let's deal with some specifics though (related to your code).
You are using 'extends DialogFragment' to create an Alert type dialog box.
There is a standard (common) way to do this, which is detailed on this Android docs Class Overview page (that deals with the 'life cycle' of DialogFragments):
http://developer.android.com/reference/android/app/DialogFragment.html
Of course the AlertDialog section is the one you want to look at first:
http://developer.android.com/reference/android/app/DialogFragment.html#AlertDialog
A careful reading (and understanding of all the code on this DialogFragment page)
will probably clue you in to the answer to your question:
How does the type of dialog in main activity match with the type of dialog that is being returned from the AlertDialogFragment??
Here's one article/tutorial that might be helpful to look over (since the Android docs can be a bit dry and don't always explain things much):
http://www.intertech.com/Blog/programmatically-working-with-android-fragments/
I also found this article helpful
(when I was designing a dialog that used multiple buttons and had to pass the data back to the Activity using 'AlertDialog.Builder' and 'setOnEditorActionListener'):
https://github.com/codepath/android_guides/wiki/Using-DialogFragment
Hopefully reading through all of the above links
should help increase your understanding a little.
In regard to the use of Callbacks with AlertDialogFragment type dialogs..
Personally I don't recommend this approach
but in one of the 'MovieActivity.java' objectives from one of the Android courses it's required to pass:
The reason I don't recommend callbacks?
I have seen them sometimes used by Android newbies (that know just enough to be dangerous)
to try to communicate between different UI fragments:
http://stackoverflow.com/questions/13733304/callback-to-a-fragment-from-a-dialogfragment
As it says it says at the end --don't do this!
The right way (per this page):
http://developer.android.com/training/basics/fragments/communicating.html
Often you will want one Fragment to communicate with another, for example to change the content based on a user event. All Fragment-to-Fragment communication is done through the associated Activity. Two Fragments should never communicate directly.
The page also goes on to explain how to build a Fragment to Activity (programmatic) 'interface' that allows communication (message passing) between them.
It's not the usual way to deal with AlertDialogFragments, but it's helpful in other UI situations.
One final note, though..
You haven't shown (in your code snippets above) your Android 'imports' line of code.
This is important (and could vary).
Please see Answer 1 in this StackOverFlow thread for import examples:
http://stackoverflow.com/questions/24274140/conflict-in-using-getfragmentmanager-android