Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial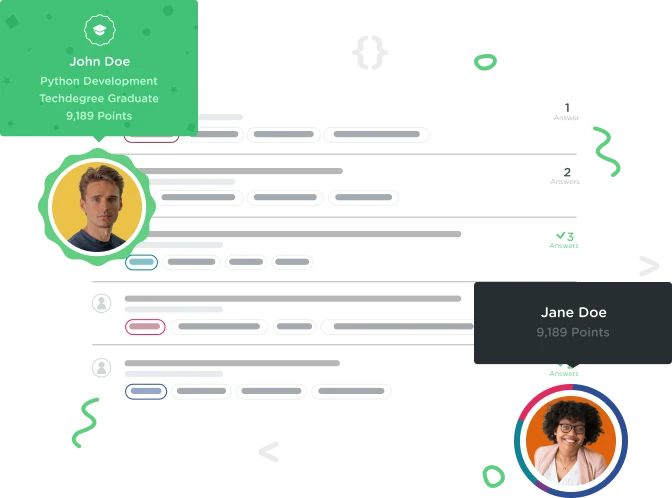
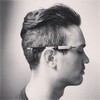
Jacob McLaws
1,497 PointsFriends not displaying on Ribbit App
There's another thread about this, but it didn't really get to the root of the problem I'm having.
Here it is: The friends list doesn't seem to respond to the friendsrelation query. I can add new friends, but they don't show up. I have followed the vid twice and still have the same issue. friendsViewController.m
#import "FriendsViewController.h"
@interface FriendsViewController ()
@end
@implementation FriendsViewController
- (void)viewDidLoad
{
[super viewDidLoad];
self.friendsRelation = [[PFUser currentUser] objectForKey:@"friendsRelation"];
PFQuery *query = [self.friendsRelation query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if(error){
NSLog(@"Error %@ %@", error, [error userInfo]);
}
else {
self.friends = objects;
[self.tableView reloadData];
}
}];
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return [self.friends count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *) indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
PFUser *user = [self.friends objectAtIndex:indexPath.row];
cell.textLabel.text = user.username;
return cell;
}
////In a storyboard-based application, you will often want to do a little preparation before navigation
//- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender { // Get the new view controller using
// [segue destinationViewController];// Pass the selected object to the new view controller.
//}
@end
Any help would be hugely appreciated.
3 Answers

Stone Preston
42,016 Pointsthe problem could be the fact that you are running the query in view did load. this only runs one time, when the view first gets loaded. So it runs when your first open the friends list, then you add some friends and navigate back to the friends list and the query does not run again so you dont see it update. try moving the query code into viewWillAppear:
-(void) viewWillAppear: (BOOL) animated
{
[super viewWillAppear:animated];
self.friendsRelation = [[PFUser currentUser] objectForKey:@"friendsRelation"];
PFQuery *query = [self.friendsRelation query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if(error){
NSLog(@"Error %@ %@", error, [error userInfo]);
}
else {
self.friends = objects;
[self.tableView reloadData];
}
}];
}
if that does not work, verify that the friends are actually being added using the parse databrowser and double check that your relation is actually named "friendsRelation". you may have mistyped it or something
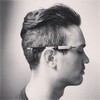
Jacob McLaws
1,497 PointsYou're on fire Stone Preston. Thanks. I wonder why Ben's was set up with View Did Load..
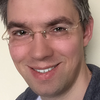
Thorsten Herbst
11,935 PointsYou can do it also in ViewDidLoad. I think you have to... because Relation has to be loaded for the first time.
Erion Vlada
13,496 PointsErion Vlada
13,496 PointsUsing viewWillAppear will mean you are sending a lot more request then you need to the server. If you had a lot of users then it is not a good idea.
You should look into NSNotification, basically like a radio and receiver type system. When you add a friend, the radio would transmit, “I HAVE JUST ADDED A FRIEND” to which your FriendViewController (the receiver) would be listening in to that station which can then execute a method to update the list of your friends.
This is great because you will only be asking the server for information when you actually need it, instead of every single time the user goes from one view, then back to your Friends view.
Its not difficult to implement, here is a link to help you: http://stackoverflow.com/questions/2191594/send-and-receive-messages-through-nsnotificationcenter-in-objective-c
Hope this helps :)