Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial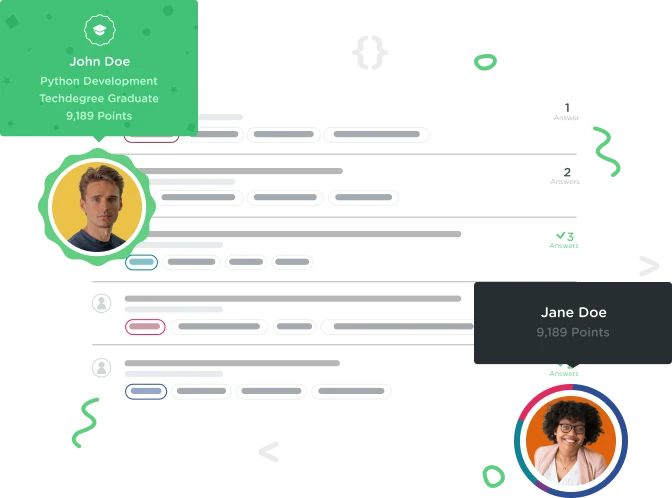

heatherglasier
2,011 PointsFrog and bird can move before space bar is pressed.
using UnityEngine; using UnityEngine.UI; using UnityEngine.SceneManagement;
public class gameState : MonoBehaviour {
private bool gameStarted = false;
[SerializeField]
private Text gameStateText;
[SerializeField]
private GameObject player;
[SerializeField]
private BirdMovement birdMovement;
[SerializeField]
private FollowCamera followCamera;
private float restartDelay = 3f;
private float restartTimer;
private PlayerMovement playerMovement;
private PlayerHealth playerHealth;
// Use this for initialization
void Start() {
Cursor.visible = false;
playerMovement = player.GetComponent<PlayerMovement>();
playerHealth = player.GetComponent<PlayerHealth>();
//Prevent the player from moving at the start of the game
playerMovement.enabled = false;
//Prevent the bird from moving at the start of the game
birdMovement.enabled = false;
//Prevent the follow camera from moving to its game position
followCamera.enabled = false;
}
// Update is called once per frame
void Update() {
//If the game is not started and the player presses the space bar...
if (gameStarted == false && Input.GetKeyUp (KeyCode.Space)) {
//...then start the game
StartGame();
}
//If the player is no longer alive...
if (playerHealth.alive == false) {
//...then end the game...
EndGame();
//...increment a timer to count up to restarting...
restartTimer = restartTimer + Time.deltaTime;
// ...and if it reaches the restart delay...
if (restartTimer >= restartDelay) {
// ...then reload the currently loaded level.
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
}
}
private void StartGame() {
// Set the game state
gameStarted = true;
// Remove the start text
gameStateText.color = Color.clear;
// Allow the player to move
playerMovement.enabled = true;
//Allow the bird to move.
birdMovement.enabled = true;
//Allow the camera to move
followCamera.enabled = true;
}
private void EndGame() {
gameStarted = false;
//show the game over text.
gameStateText.color = Color.white;
gameStateText.text = "Game Over!";
//Remove the player from the game
player.SetActive(false);
}
}
1 Answer

Henrique Vignon
Courses Plus Student 6,415 PointsMake sure both your PlayerMovement and BirdMovement scripts are named exactly like that, capitalization and all.
Oh and don't forget to drag the bird object to the GameState script in the field "Bird Movement"
edit: And drag the player object in the respective field as well.

Henrique Vignon
Courses Plus Student 6,415 PointsWell, in reality you can name anything whatever you please, but it's considered best practices to capitalize the first letter for name spaces, classes, methods and attributes, then having it lower case (but still using camel case) for objects and variables.
Notice the part were you declare the player movement:
private PlayerMovement playerMovement;
In this line you're declaring a variable by the name playerMovement which is of type PlayerMovement, in unity basically each script is what we call a class (which can be also be called a type).
and then you tell the playerMovement variable you just made that it is copy of the main PlayerMovement, that in turn is in the player object, so you can control it:
playerMovement = player.GetComponent<PlayerMovement>();
So unless the PlayerMovement component that is in the player object has that exact name, it will not find it in order to assign it to the playerMovement variable in this script.
I'm not too good with explaining things :) but I suggest you do the C# basics course to understand a bit more about the scripting side of it (if you're following the game development track it's a bit later in it), or just re-watch the videos in which the teacher shows how to write those scripts.
heatherglasier
2,011 Pointsheatherglasier
2,011 Pointsokay but don't you have to have it lowercase at the start of some of the PlayerMovement and BirdMovement scripts so like playerMovement instead of PlayerMovement