Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial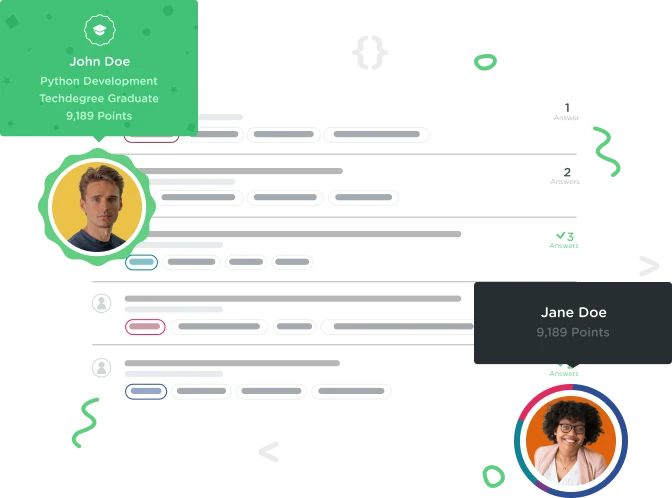
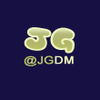
Jonathan Grieve
Treehouse Moderator 91,253 PointsFrog can move before the Spacebar is pressed
Hello all,
I'm almost there with the game. I can play the game fully and the game states work, but when testing the game, I can see that the frog can be moved before the space bar is pressed and of course the camera doesn't follow it until I do. I'm pretty sure what's not supposed to happen.
There were a couple of Red errors in the console but those have now disappeared.
I know that everything else is working because the bird doesn't chase the frog until the spacebar is pressed even after the first go and the game is back in the Start State.
Here's my code. :)
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameState : MonoBehaviour {
private bool gameStarted = false;
[SerializeField]
private Text gameStateText;
[SerializeField]
private GameObject player;
[SerializeField]
private BirdMovement birdMovement;
[SerializeField]
private FollowCamera followCamera;
private float restartDelay = 3f;
private float restartTimer;
private PlayerMovement playerMovement;
private PlayerHealth playerHealth;
// Use this for initialization
void Start () {
Cursor.visible = false;
playerMovement = player.GetComponent<PlayerMovement> ();
playerHealth = player.GetComponent<PlayerHealth> ();
//prevent the player from moving tbatth start of the game
playerMovement.enabled = false;
//prevent the bird from moving at the end of the game
birdMovement.enabled = false;
//prevent the follow camera from moving into its game position
followCamera.enabled = false;
}
// Update is called once per frame
void Update() {
//If the game is not started and the player presses the space bar...
if(gameStarted == false && Input.GetKeyUp(KeyCode.Space)) {
//start the game
StartGame();
}
if(playerHealth.alive == false) {
//end the game
EndGame();
//increment a timer to count up to restarting
restartTimer = restartTimer + Time.deltaTime;
//..and if it reaches the restart delay...
if(restartTimer >= restartDelay) {
// ...then reload the currently loaded level
//Application.LoadLevel(Application.loadedLevel);
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
}
}
private void StartGame() {
//set the game state
gameStarted = true;
//Remove the start text
gameStateText.color = Color.clear;
//Allow the player to move
playerMovement.enabled = true;
//Allow the bird to move
birdMovement.enabled = true;
//Allow the camera to move;
followCamera.enabled = true;
}
private void EndGame() {
//set the game state
gameStarted = false;
//Show the game over text
gameStateText.color = Color.white;
gameStateText.text = "Bad Luck! Game over";
//Remvoe the player from the game
player.SetActive(false);
}
}