Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial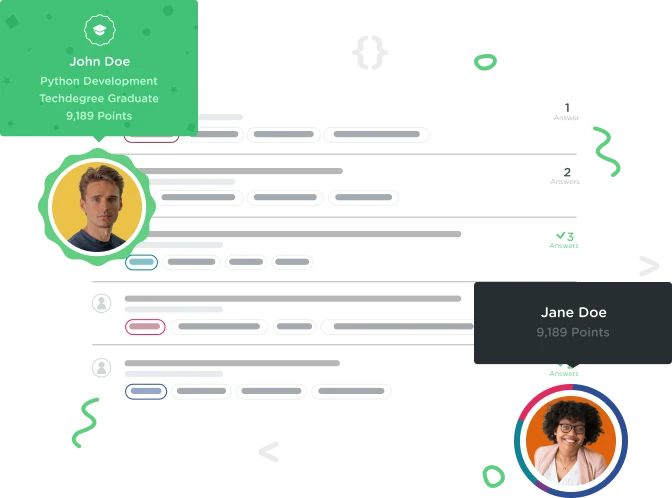

aaron terry
644 PointsFrog won't move. I believe my code is correct
Here is my code:
using UnityEngine; using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator>();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Veritcal");
movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate ()
{
if (movement != Vector3.zero)
{
playerAnimator.SetFloat("Speed", 3f);
}
else
{
playerAnimator.SetFloat("Speed", 0f);
}
}
}
4 Answers

edwinshen
13,168 PointsYou probably did not attach the script onto the player. If your code is correct, go to the Hierarchy, click Player. Then go to inspector on the right and make sure you added the PlayerMovement script. If you didn't just press add component --> Scripts and then click playermovement.

Helen Burrows
5,786 PointsThank you so much Edwin, I've been reading and re-reading my code for hours trying to work out why the frog wasn't moving. Your advice solved my problem :)

edwinshen
13,168 PointsNo problem Helen! I had the same problem and I didn't want others to waste their time figuring it out. Glad it helped.
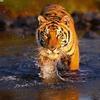
Konrad Pilch
2,435 PointsI dont know, but you believe is corrrect, what i woudl do, is to downolad the code and past this page and see if it will move.
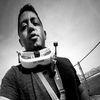
hector villasano
12,937 Pointscheck spelling and punctuation !
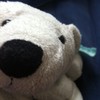
bothxp
16,510 PointsHi Aaron,
Just in case you were still stuck on this, you need to look at the line
moveVertical = Input.GetAxisRaw ("Veritcal");
You have misspelled the name of the input axis, so you just need to change it to "Vertical". I make that mistake myself all of the time.
aaron terry
644 Pointsaaron terry
644 PointsForgot to mention that unity is giving me this error: ArgumentException: Input Axis Veritcal is not setup. To change the input settings use: Edit -> Project Settings -> Input PlayerMovement.Update () (at Assets/Scripts/PlayerMovement.cs:19)