Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial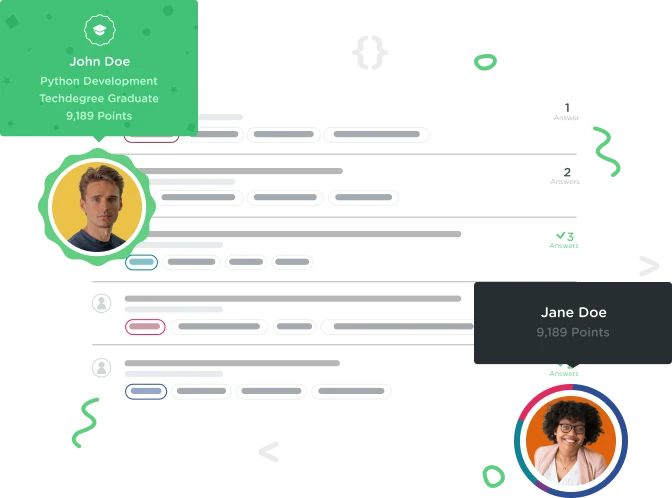
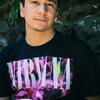
Kyle Calica
5,955 Pointsfrom_string: A weird issue where b is 'dot' won't run conditional but b == 'dot' will?
I have this weird issue where my code works with ==
in the if
statements but not with is
but I've used this is
before for check '.' and '_' in an earlier answer:
"-".join(['dot' if p is '.' else 'dash' if p is '-' else '' for p in pattern].strip(''))
Even prints out both are true. Do I have some error in my format? I want to get this to another one-liner using is
as it's more pythonic that way.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, string):
#return cls( [ '.' if b is 'dot' else '_' if b is 'dash' else '' for b in string.lower().split('-') ] )
blips = string.lower().split('-')
marks = []
for b in blips:
if b is 'dot':
marks.append('.')
if b is 'dash':
marks.append('_')
return cls(marks)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer
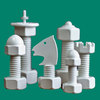
Steven Parker
230,274 PointsActually, that's not a proper use of "is". The "==" operator is a test for value equality, which is the correct one for this situation. The "is" operator is a test for identity.
The Identity test only worked because of a technicality in the way Python stores string literals for efficiency, definitely not something that the code should rely on!