Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial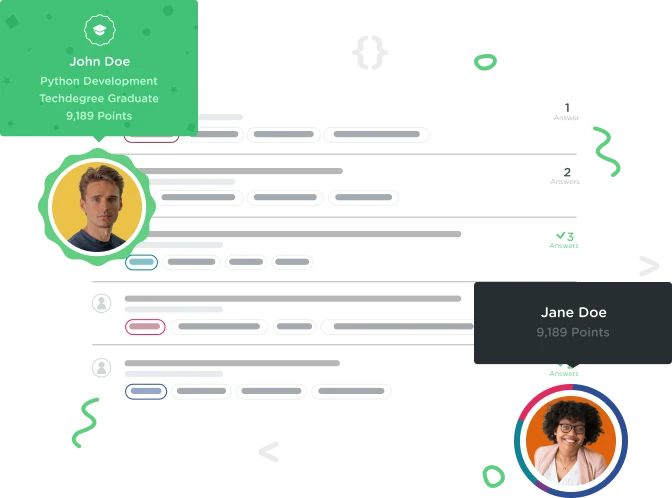
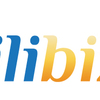
Mathieu Martinot Dubary
4,978 PointsFunction and length return
Hi again,
I must be very stupid but I don't understand my mistake for this one.
"Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in"
<script>
function arrayCounter (name) {
if (typeof name === 'undefined', 'string', 'number') {
name = "0";
}
return name.length;
}
arrayCounter();
</script>
Instead of having "0" if name is undefined, I have 1. Actually I have "1" all the time, even when I put a real name in arrayCounter.
Thank you for your help.
5 Answers
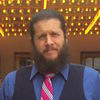
Adam Moore
21,956 PointsYou have to use the "or" operator to compare, as you are attempting to check to see if the argument "array" is a "typeof" a "string" OR a "number" OR an "undefined" value.
var arrayCounter = function(array){
if(typeof array == 'string' || typeof array == 'number' || typeof array == 'undefined'){
return 0;
}
return array.length;
}
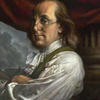
Jeff Busch
19,287 PointsHi Mathieu,
This one seems to give a lot of people trouble, myself included. Your if condition needs to be more explicit.
Jeff
function arrayCounter(array) {
if (typeof array === "string" || typeof array === "number" || typeof array === "undefined") {
return 0;
} else {
return array.length;
}
}
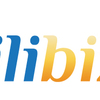
Mathieu Martinot Dubary
4,978 PointsThank you Jeff

alex mattingley
7,508 PointsHey I know this has already been answered, but I thought I might try to contribute a bit more. At this point in my javascript learning I was actually unaware of the 'or' operator which is the || sign above. Another way to do it without the or operator is:
function arrayCounter(array) {
if (typeof array === "string") {
return 0;
}
if (typeof array === "number") {
return 0;
}
if (typeof array === "undefined") {
return 0;
} else {
return array.length;
}
}
I think the or operator is probably more correct, but this works too, and looking at both sets of code helped me to understand everything a little bit better.

Jason Anello
Courses Plus Student 94,610 PointsHi Alex,
It's not so much that it's more correct but it's more readable and it removes the repetition. You don't have 3 return statements all returning the same thing.
Your solution here is about what would be expected based on what you've learned so far. It would be hard to come up with the OR solution without doing some research on your own.

kudusdabiri
Courses Plus Student 11,578 PointsHi Mathieu, If you are trying to count the lenght of an array maybe you should check that your arguement is actually an array instead of checking that it's not.
Try this:
function arrayCounter(name) {
if (Array.isArray(name)) {
return name.length;
} else {
return name = 0;
}
}
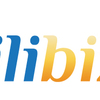
Mathieu Martinot Dubary
4,978 PointsThank you for your answer Kudus
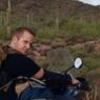
Kevin Naegele
10,868 PointsYeah they do not talk about the OR function before this challenge. I think the instructor wanted us to use Alex's way, but the OR function does pass the challenge as well.
Mathieu Martinot Dubary
4,978 PointsMathieu Martinot Dubary
4,978 PointsThank you a lot it's working :)