Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial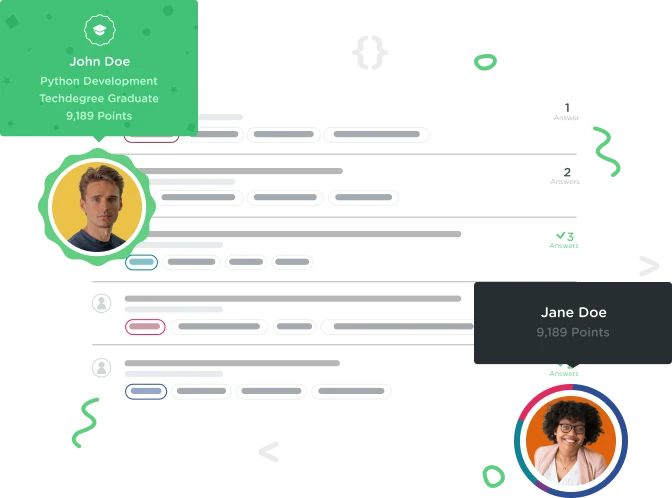

Ramesh Kunwar
Courses Plus Student 4,066 Pointsfunction as parameter
I am not understanding anything about function as parameter
1 Answer

Cooper Runstein
11,850 PointsThe explanation given in the video is pretty hard to understand, I struggled to understand what was going on when I first saw this one too. Here's an example of functions passed as parameters:
const double = function(number){
return (number * 2)
}
const addTwoThenDo = function(number, func){
let newNum = number + 2;
return func(newNum);
}
addTwoThenDo(5, double) //this will return 14
addTwoThenDo(3, double) //this will return 10
Above is an example of how passing a function to another function works: the function double is pretty self explanatory, but addTwoThenDo is a function that takes a callback. addTwoThenDo accepts a number and a function. addTwoThenDo will add 2 to the number it is given and sets that equal to a variable newNum, then when that's done, it calls back the function passed to it, and runs the new value calculated from the initial number through the function that was passed to addTwoThenDo. Notice how the function is passed in without the parentheses, that is because the function isn't going to be called until addTwoThenDo decides to call it, which happens after its work is done.
This allows for a lot of really cool things that you'll likely pick up later, like the map, filter and reduce functions in ES6, but in the case of the video, it allows for the window.setTimeout function.
const myFunction = function(){
...do cool things
}
windom.setTimeout(myFunction, 3000) #myfunction is the callback, it's set to run after setTimeout does its work, which in this case is to wait for 3000MS.
Hopefully this helps some, you're going to deal with this concept a lot in the coming videos, so if you stick with it I'm sure it'll make sense.
Daniel LeaMon
5,127 PointsDaniel LeaMon
5,127 PointsThanks this helped a lot!