Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial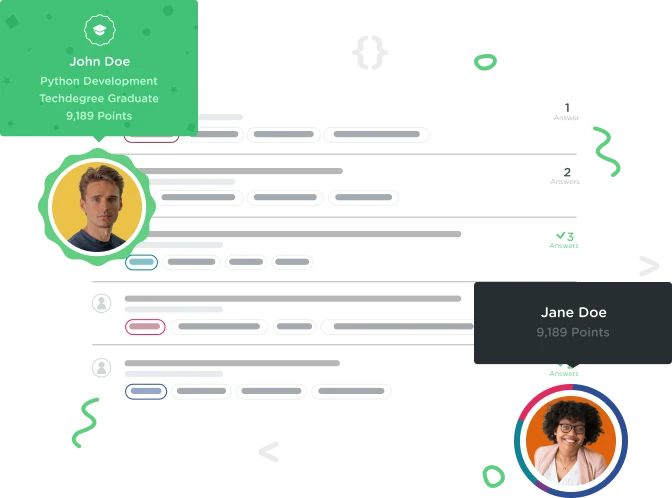
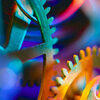
ja5on
10,338 Pointsfunction breakdown problems
function exec(func, arg) {
func(arg);
}
exec((something) => {
console.log(something);
}, "Greetings, everyone!");
Can someone break this down step by step please
2 Answers

babyoscar
12,930 Pointsfunction exec(func, arg) {
func(arg);
}
defines a function called "exec". It has two parameters. The first one, func
, is supposed to be a function that accepts one parameter. The second one, arg
, is the argument you pass to func
. Inside "exec", it calls func
and passes arg
as the first parameter.
exec((something) => {
console.log(something);
}, "Greetings, everyone!");
calls "exec", and passes a function for the first argument to "exec". This function accepts one argument (as expected). It logs that argument to the console. It passes "Greetings, everyone!" as the second argument to "exec". "exec" calls the function that was passed as the first argument to "exec", passing "Greetings, everyone!" as the argument. So, the call to "exec" basically executes console.log("Greetings, everyone!");
. Hope this helped!
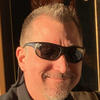
Peter Vann
36,427 PointsHi jas0n!
It might be easier to grasp if written more like this:
function exec(func, arg) { // When exec is called...
func(arg); // ...this function will execute (which, as coded, is actually logGreetingFunc)
}
const logGreetingFunc = (something) => {
console.log(something);
}
exec(logGreetingFunc, "Greetings, everyone!"); // Actual execution happens here
When exec runs, this will log to the console:
Greetings, everyone!
It may seem silly or weird to pass a function to a function and in this case, there is no real benefit over just doing this:
const logGreetingFunc = (something) => {
console.log(something);
}
logGreetingFunc("Greetings, everyone!");
But there are cases where, say for timing reasons, you want to delay the execution of logGreetingFunc, you can pass it to another function that handles the delay, such as setTimeout or addEventListener and it will execute either after the delay or when the event occurs (such as a button click).
More info:
https://teamtreehouse.com/library/what-is-a-callback
https://www.freecodecamp.org/news/what-is-a-callback-function-in-javascript/
Think of exec as a sort of wrapper function (much like decorators in Python) and it could even contain some helper code, for example, perhaps validating "something" before passing it to logGreetingFunc. Or exec could return True or False depending on if logGreetingFunc's execution is successful or not.
More info:
https://trackjs.com/blog/how-to-wrap-javascript-functions/
https://teamtreehouse.com/library/understanding-closures-in-javascript
I hope that helps.
Stay safe and happy coding!
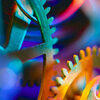
ja5on
10,338 PointsOk thanks Peter! I took the code from one of the dom videos, I'm glad to say I have made it through four stages out of five! So I well pleased lol... I decided to stick with it. :-)