Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial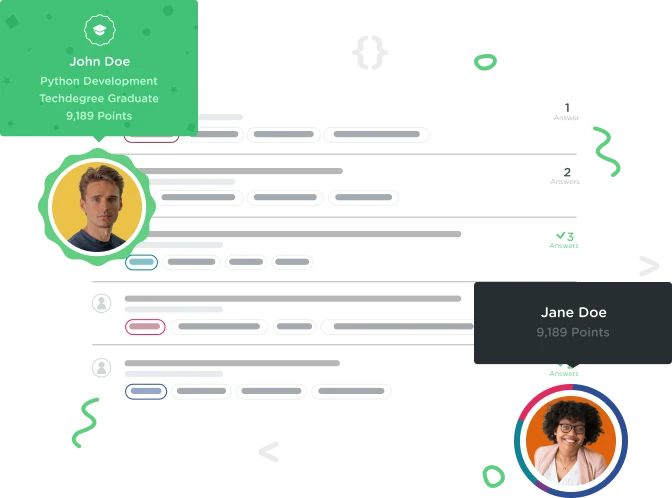

Steve Muthig
4,225 PointsFunction Challenge Help
Can anyone point me in the right direction with this challenge
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
Bummer! Wrong value returned from 'arrayCounter(undefined)' it should be 0
function arrayCounter (my_array) {
var my_array = [1,2,3];
if (my_array === 'undefined')
return 0;
arrayCounter.length;
}
5 Answers

Art Skupienski
7,080 PointsHi Tyler,
I see your point to simplify the code which is in most cases good way. However there are some syntax rules we need to follow. Whenever you pass conditions to your if statement you need to be more specific. Otherwise your code may not be executed.
Add typeof array ===
before 'string' and also before 'number' and you will be ready to go :-) Keep going and have fun with Javascript!
Just like below:
function arrayCounter(array) {
if (typeof array === 'undefined' || typeof array === 'string' || typeof array === 'number')
return 0;
else
return array.length;
}

Art Skupienski
7,080 PointsYou can try to rewrite this code using shorter way too.
variable = (condition) ? true-value : false-value;
However keep in mind when you simplify your code automatically it becomes less readable especially when is much more complex and it's for plugin development.

Art Skupienski
7,080 PointsHi Steve,
In order to accomplish this you will need yo create function called 'arrayCounter' like this:
function arrayCounter(){
}
Now you need to pass a parameter which will be an array, however you do not need to create variable here. It can be any parameter name which you will then use inside this function. Let's say you array will be 'a':
function arrayCounter(a){
}
Now inside this function you have to use if statement to check if any argument you pass to function is a string or namer, or undefined. In order to defined type of your a parameter you passed in you use 'typeof':
function arrayCounter(a){
if(typeof a === 'string' || typeof a === 'number' || typeof a === 'undefined'){
return 0;
}
return a.length;
}
Best of luck with your Javascript course!
Art

Tyler Malone
7,424 PointsHi Art,
Why does this similar way not work?:
function arrayCounter(array) {
if (typeof array === 'undefined' || 'string' || 'number')
return 0;
else
return array.length;
}
Thanks!
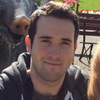
Justin Iezzi
18,199 PointsTyler,
Try
function arrayCounter(array) {
if (typeof array === ('undefined' || 'string' || 'number'))
return 0;
else
return array.length;
}
The way you have it written is only checking if array === 'undefined', for it to work without the parenthesis you would need
(typeof array === 'undefined' || typeof array === 'string' || typeof array === 'number')

Steve Muthig
4,225 PointsThanks for the info Art!

Steve Muthig
4,225 PointsThanks for all the replies!
Steve
Tyler Malone
7,424 PointsTyler Malone
7,424 PointsThanks Art! I was not aware if statements needed such specificity.