Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial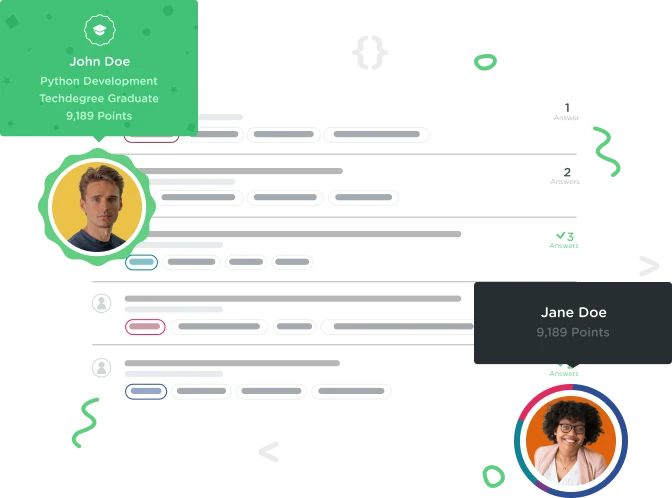

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsFunction code challenge:How to do total
I am trying to do total, as the quiz says but i am doing something wrong in the function,, following is my code
function mimic_array_sum($array){
foreach ($array as $element){
$count = $element + 1;
}
return $count;
}
$palindromic_primes = array(11, 757, 16361);
$count = mimic_array_sum($palindromic_primes);
echo $count;```
That is case of adding one, works fine but what it says in quiz is you need it to have add the values. how can i add $element + $elements ?
4 Answers

banned banned
Courses Plus Student 11,243 PointsShouldn't you define the count variable outside of the foreach loop? Now it will over-run count in the loop.
So you would get someting like this:
function mimic_array_sum($array){
$count = 0;
foreach ($array as $element){
$count .= $element + 1;
}
return $count;
}
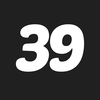
Casey Morris
8,003 PointsThe way you have wrote this, it would only echo and return the last number in an array plus 1. With the way you currently have it written, each time the loop iterates, the previous number is lost as you are not adding in the previous total. What you need to be doing is getting a running total of the numbers in the foreach loop. This can be done by adding the count to itself and the element that needs to be added. By doing this, you will get the sum of all numbers in the array.
Edit: The above answer still would not give a sum of all numbers in the array, but simply return the last number in the array with 1 added to it. Each iteration of the loop would simply reset the value of $count to the current element with 1 added to it. You also do not need to define $count outside of the loop as it is redundant. In the loop, you need to set the value of $count to itself added to the current $element. This would look something like this:
$count = $count + $element;

Jared Adamson
2,896 PointsI believe the question was to create a function that added up the sum of all the values in the array. You can do by running through the foreach loop adding the next $element value to the $count variable.
function mimic_array_sum($array){
foreach ($array as $element){
$count = $count + $element;
}
return $count;
}
This is basically is setting a variable called $count and adding each value $element each time to get the total sum.
So the first time the loop runs:
$count = 0 and the $element has a value of 11 which is added to $count and stored in the variable $count.
The second loop:
$count now has the value of 11 which is added to the next $element value of 757 and stored in the variable $count.
The third loop;
$count = 768 + $element = 16361 and is stored back in the variable $count.
The statement:
$count = $count + $element;
[$count + $element] is calculated and then is stored in the variable $count.

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsIt is confusing to make a $variable based function, which later do what is been said in function, can someone explain?

Jared Adamson
2,896 PointsAre you confused about the scope of the variable (difference between global variables and variables used in the function)?
Or
A variable storing the return value of the function like:
$myvariable = MyFunction();
Or
how to pass a variable made earlier outside the function inside the function?