Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial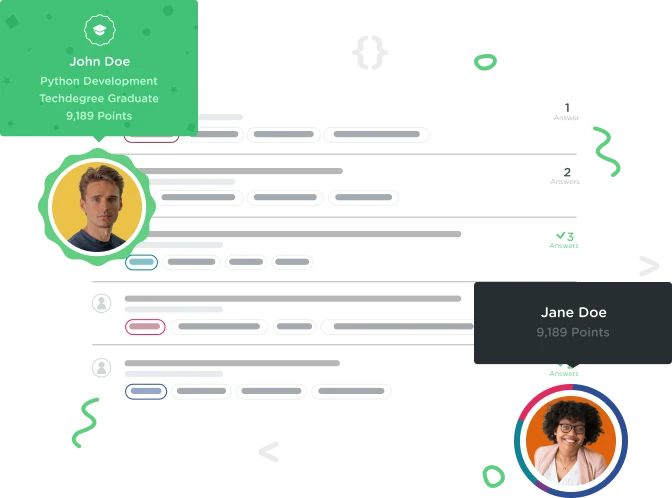

Derick Brown
7,361 PointsFunction createLI not an arrow function?
In this video, Guil created a function called createLi:
function createLI() {
}
But in the earlier videos, he created arrow functions.
How come he didn't use an arrow function for "createLI" but instead used the older way of creating functions?
4 Answers
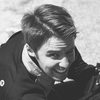
Brandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsGreat question.
When declaring a function, you'll see something like this:
function createLi() {
}
While the function declaration above is syntactically a statement, functions can also be created by a function expression. >Such a function can be anonymous; it does not have to have a name:
When using arrow functions, the function does not have a name (it's always anonymous):
ul.addEventListerner('click', (e) => {
});
This is a great resource that explains functions in greater detail: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Functions
Hope this helps!
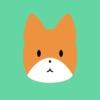
Mitul Patel
24,890 PointsTo add. Named function declarations
can be useful when debugging errors. The console will show the function name instead of stating anonymous
. This log is what is referred to as the stack trace
To cover another difference between the two involves a topic called hoisting
.
A function declarations
is hoisted making it available in within the scope. A function expression
will only be available after the assignment.
Example of a function declarations
:
function createLI() {
// Magic
}
Example of a function expression
:
let createLi = () => {
// Magic
}
For more information on hoisting
:
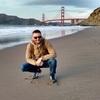
Marko Nestorovic
12,441 PointsIf you are going to use function just once, especially for event handler, you don't have function name. But if you are going to reuse it later on, you have to name your function.

Daniel Phillips
12,632 PointsFor this function, you could write the createLI
function like this:
const createLI = text => {
}
Derick Brown
7,361 PointsDerick Brown
7,361 PointsThanks for the link and info!
So you will basically only use arrow functions when you're writing function expressions. Correct?
Abraham Juliot
47,353 PointsAbraham Juliot
47,353 Points"When using arrow functions, the function does not have a name (it's always anonymous):"
Actually, arrow functions can have names.
Brandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsBrandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsAbraham Juliot, I can see what you're saying. But what it looks like you're doing is assigning an anonymous (arrow function in this case) to a named variable. So the function is assigned to the variable.
This is a direct quote from the Mozilla site on arrow functions:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Functions
Abraham Juliot
47,353 PointsAbraham Juliot
47,353 PointsHi Brandon, true, I see what your saying.