Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial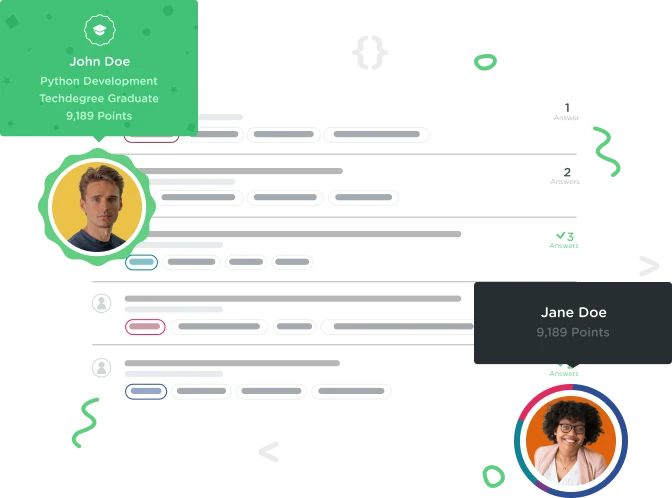
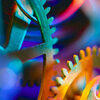
ja5on
10,338 Pointsfunction declarations vs function expressions
I am so confused Seems hard for me to know when to use one or the other and what either mean??
I need some simple programs in both to see whats happening.
1 Answer
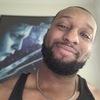
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi jasonj7,
So a function declaration goes like this:
function square(num) {
return num * num
}
And a function expression goes like this:
const square = (num) => num * num
As you can see in the code above, function expressions occasionally allow you to write code that is shorter. But the real difference is that function declarations are hoisted, while expressions are not. This means that even if your function isn’t declared until line 327, you can still call the function on line 5, because the JavaScript interpreter basically looks for all those declarations first and remembers them.
If you tried to invoke a function defined through a function expression before the expression had been encountered in code, you’d get a variable “name-of-function” not defined error.
Does that make sense?
Hopefully it does and that helps to guide you on when to use one versus the other, but a lot of the time it’ll be your personal preference on which version to use.
ja5on
10,338 Pointsja5on
10,338 PointsI find declarations are easier to read, I tend to go for more readable code (less confusing). So function declarations can be called anywhere within the program, but function expressions have to be placed where they are expected to be? Sorry if I'm repeating what your saying..
So then would it be easier to use declarations? But then expressions are stored in variables so I'm confused as to what would be more useful, so would it depend on the program your creating and its requirements?
I think I'm struggling to see how function Expressions work?
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsUm... program can be such a broad term. I wouldn’t even know how to play all the potential use cases in my head, so I don’t want to say that you can call a function defined within a declaration anywhere within a program. In my mind, a program can consist of infinite many files.
A function defined within a declaration can be called anywhere within that same file. I think that’s a safer way to state it.
But yes, function expressions have to be stated before their resulting functions can be called/invoked.
I tend to use expressions more. They tend to allow me to write less code, and I appreciate the structure they force. While it’s important to understand hoisting, it’s a good practice to place your functions at the top of your file (and not just anywhere—which you can still do with declarations). Although it doesn’t make a real difference to the machine that’s executing the code, it makes a huge difference to the programmer who’s going through the code and trying to understand it.
The truth is one is not MORE useful than the other in a general sense. There are times where you will want to use an expression because it allows you to use arrow syntax or because you want/need your function to be stored in a variable. There are other times where you’ll want/need your function to be a declaration.