Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial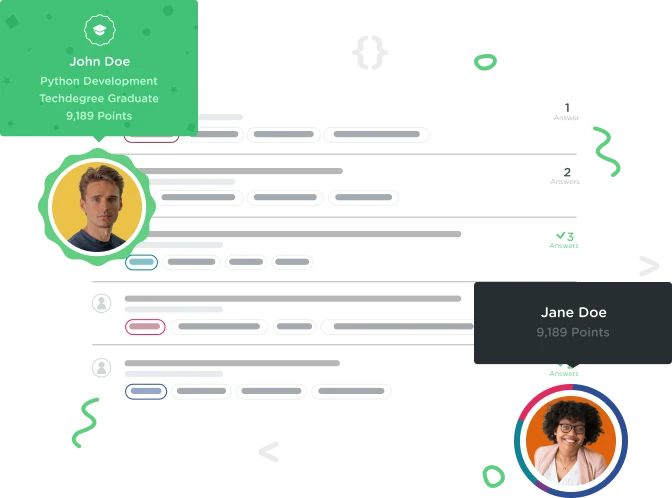

Kip Yin
4,847 PointsFunction default parameter values
I am super confused about the rules on default parameter values in JavaScript.
Say I have a function:
function foo(a=1, b=2, c=3) {
return a + b + c;
}
When I run foo(a=2, b=3, c=4)
in different places I got different results.
- In the command line
node.js
(v8.9.1
) and Safari console, this is perfectly fine. - In Atom, with
script
package (which also usesnode
to run scripts), I got a reference error, saying thata
is not defined.
Is this just an Atom-specific issue? And what is the best practice to set and use default parameter values?
1 Answer
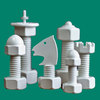
Steven Parker
231,007 PointsThe equal signs in the function definition establish default values, but using them in the function call does something entirely different. This would create or reassign global variables, which is probably not what you are intending. It can also cause an error if it is being run in "strict" mode.
So instead of "foo(a=2, b=3, c=4)
", you should just call it with "foo(2, 3, 4)
". The defaults only take effect when you leave off one or more arguments (such as "foo(5, 6)
" or "foo()
").
Kip Yin
4,847 PointsKip Yin
4,847 PointsThanks for replying. What if I want to skip a parameter? Say I want to set
a=5
andc=6
while using the default value ofb
?Steven Parker
231,007 PointsSteven Parker
231,007 PointsYou'd need to pass the parameters in a different way. For example, using an object:
Happy coding!
Kip Yin
4,847 PointsKip Yin
4,847 PointsFantastic. Thank you!