Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial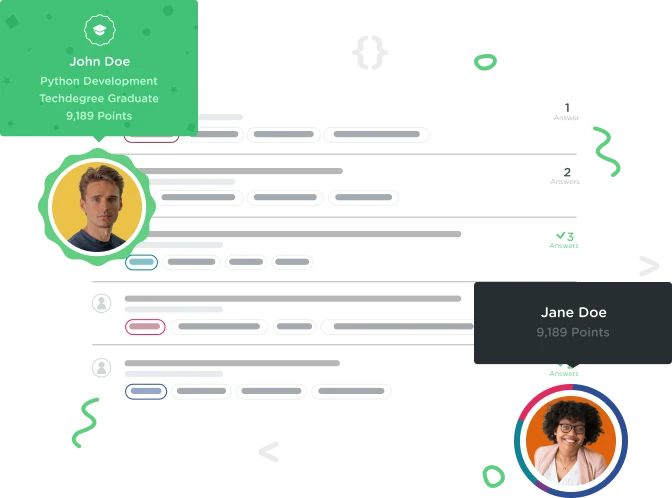

Wojciech Ratajczak
1,925 PointsFunction delete item - Raise ValuError
I tried to add a new function to remove an item from the list. It's working ok, until I enter an item, which is not on the list. Can anyone help how to use ValueError properly?
shopping_list = []
def show_help():
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to show the list.
Enter 'DEL' to remove an item from the list.
""")
def add_to_list(item):
shopping_list.append(item)
print("{} added to the list".format(item))
print("now you have {} item(s) on the list".format(len(shopping_list)))
def show_list():
print("Here is your list:")
for item in shopping_list:
print(item)
def del_item(item):
try:
item = input("Which item do you want to remove?")
if item not in shopping_list:
raise ValueError("You don't have it on the list.")
except ValueError as e:
print("Try again. {} ".format(e))
shopping_list.remove(item)
print("{} removed from the list".format(item))
show_help()
while True:
new_item = input("> ")
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list()
continue
elif new_item == 'DEL':
del_item(new_item)
continue
add_to_list(new_item)
show_list()
3 Answers

Justin Cox
12,123 PointsHello! Good job adding a DEL function to your list, by the way!
You are super close! What's happening is, even though you are raising a ValueError
when the item is not in the list, the program finishes the try/except block, then jumps down to the end of the del_item
function (the last two lines) and tries to still remove the item anyway. Since the item doesn't exist in the list, it throws a brand new ValueError ("list.remove(x): x not in list")
Oops! So the shopping_list.remove(item)
gets run no matter what! We just need to make sure this line only runs if the item IS in the list.
All you have to do is add an else
block to your try/except block and move your last two lines inside there! Like this:
def del_item(item):
try:
item = input("Which item do you want to remove?")
if item not in shopping_list:
raise ValueError("You don't have it on the list.")
except ValueError as e:
print("Try again. {} ".format(e))
else:
shopping_list.remove(item)
print("{} removed from the list".format(item))
This reads, "try to remove (item), raise an error if it doesn't exist, ELSE remove the item (since it exists)"
I hope that helps!

Wojciech Ratajczak
1,925 PointsWow, so easy and so hard to find out for me. I really do have problems with ValueError syntax. So I've found ways to do it without it. Actually, even on my basic level there are many ways, and this is only one of them:
def del_item(item):
item = input("Which item do you want to remove?")
while item not in shopping_list:
print("{} is not on the list. Try again".format(item))
item = input("Which item do you want to remove?")
shopping_list.remove(item)
print("{} removed from the list".format(item))
Thank you very much Justin!

K1 Fa
626 Pointshey ... when i read your code and test it, i don't get this part:
if item not in shopping_list:
why we can't use this instead:
if item != shopping_list:
what is the difference?? and why when i use
if item != shopping_list:
the code doesn't work properly ??