Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial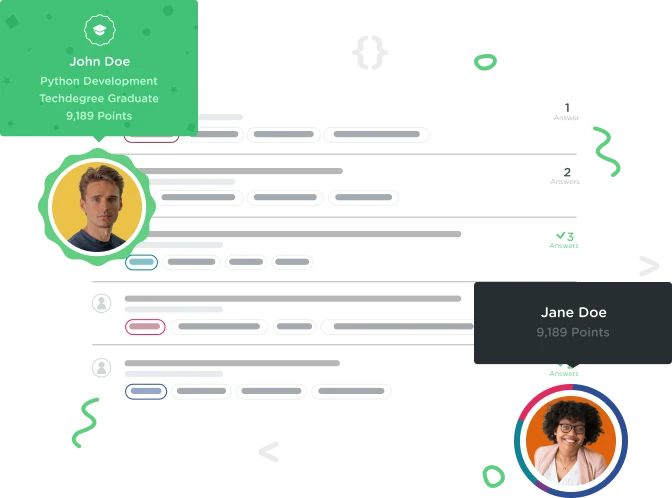

Austin March
2,310 PointsFunction help python
i dont understand how to pull the value out of function. how can i do this. im trying to complete the objective in python basics of functions. the lesson question is called "creating a function. Heres what i have so far.
1 Answer
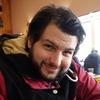
Eric M
11,545 PointsHi Austin,
I took at some of the code you linked and in your tests.py file I found your square(number_to_be_squared)
function.
Here's the good news: there's nothing wrong with this function! You've figured out functions and return values. Your function has no issues. The way that you're calling this function does have an issue though, and it's got to do with variable types.
Your square()
function is multiplying your argument by itself, so you need to pass square an argument that can be multiplied by itself. This works fine for things like ints (e.g. -5, 100, 2) and floats (e.g. 3.14, -0.12, 9999.99) but it doesn't work if you try something like wisdom = "experience" * "knowledge"
. The thing is, it also doesn't work if you try result = "5" * "10"
Strings are a sequence of characters, so they get treated very differently than numeric values.
You're getting the number to be passed to your square()
function from the input()
function. The input function returns a String!
The good news is it's very easy to convert between a string and a numeric type if the sequence of characters is digits. For the sake of example, let's assume your users are always going to type integers and only integers.
We can convert a string of numeric characters into an integer value with the int()
function. You can put your input call inside the int function like user_input = int(input("What number would you like to square? "))
or you can split it out over two lines for readability:
user_input = input("What number would you like to square? ")
user_integer = int(user_input)
However you like, then when you pass that value to your square function it should work!
If you're interested in reading more about this, this type of conversion between one datatype and another is called a "cast" or "casting" from a string to an int. Different programming languages handle this (and many other things) differently.
P.S.
While you can't multiply a string by a string, you can multiply a string by an int! If you used foo = 5 * "good luck! "
foo would then store the value 'good luck! good luck! good luck! good luck! good luck! '