Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial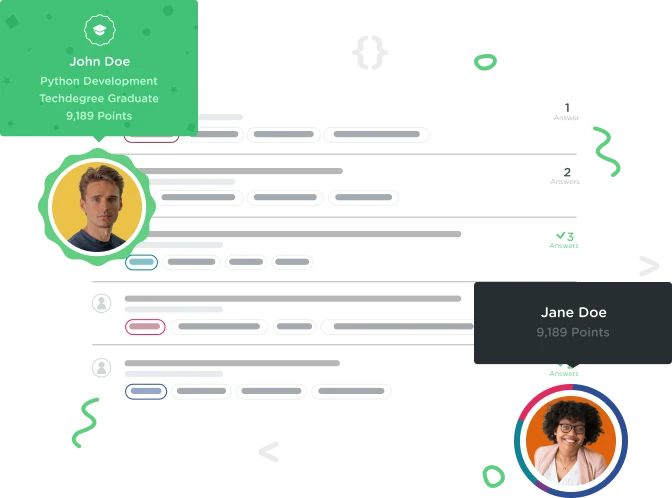

Max van den Berge
6,506 Pointsfunction logTeacher(teacher) { console.log(`${person.name} - ${teacher.role}`); }
In this course the following code is used.
const person = { name: 'Andrew Chalkley', role: 'JavaScript Teacher' }
function logTeacher(teacher) {
console.log(${teacher.name} - ${teacher.role}
); }
logTeacher(person);
Q: my question is this. from what i understand this function ' teacher' is using properties from the object literal person. in the line console.log(${teacher.name} - ${teacher.role}
); }
i would have tought that the code should be:
console.log(${person.name} - ${peron.role}
); } . both code options give the same results.
why does ${teacher.name} and ${person.name} give the same results?
1 Answer

jb30
44,806 Points${teacher.name}
and ${person.name}
give the same result in this case because logTeacher
is only called once, with the argument person
, in the line logTeacher(person);
.
If you change the argument passed into the function, the output will change.
const person = { name: 'Andrew Chalkley', role: 'JavaScript Teacher' };
logTeacher(person); // outputs 'Andrew Chalkley - JavaScript Teacher'
const person1 = { name: 'Max van den Berge', role: 'JavaScript Student' };
logTeacher(person1); // outputs 'Max van den Berge - JavaScript Student'
If you change the logTeacher
function to
function logTeacher(teacher) {
console.log(`${person.name} - ${person.role}`);
}
then the teacher
parameter will be ignored in the output:
const person = { name: 'Andrew Chalkley', role: 'JavaScript Teacher' };
logTeacher(person); // outputs 'Andrew Chalkley - JavaScript Teacher'
const person1 = { name: 'Max van den Berge', role: 'JavaScript Student' };
logTeacher(person1); // outputs 'Andrew Chalkley - JavaScript Teacher'
Max van den Berge
6,506 PointsMax van den Berge
6,506 Pointsthx jb30 for this explanation.
Is this thought process correct:
The function has an argument ( teacher ) , but the output logTeacher ( the name of the function ) refers to the object literal person1. In this object literal there is a value of