Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial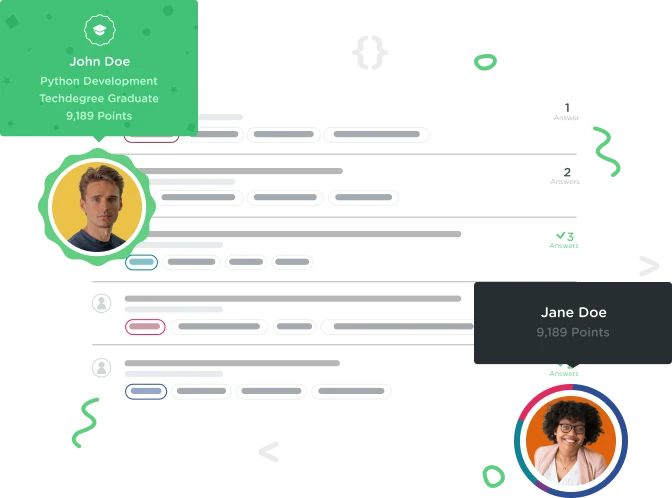
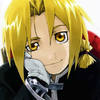
Gabriel D. Celery
13,810 PointsFunction parameter and global variable question
I have a little bit of an issue understanding how JS handles local and global variables if they have the same name.
If I type in this:
var number = 10;
function test(parameter) {
number = parameter + 2;
}
test(number);
document.write(number);
I get the desired effect and the function changes the global variable.
But if I type in this:
var number = 10;
function test(number) {
number = number + 2;
}
test(number);
document.write(number);
Then the global variable is not affected. I assume this happens because JS treats the left "number" in the line of
number = number + 2;
as a local variable since it has the same name as the parameter, but is there any way to tell JS that I want to affect the global variable, or I have to pay attention every time how I name all the stuff and write the code?
1 Answer
Igor Yamshchykov
24,397 PointsAs Jason said it is actually a bad idea to keep your data in global scope, but sometimes you might need that.
and to answer your question:
The global variable is not affected in local function, because there is a parameter with same name and function changes that parameter. You are correct with that.
If you want to modify the global variable you might use this approach
var number = 10;
function test(number) {
return number + 2;
}
number = test(number);
it will return the modified value and you can assign that value to global variable.
Also you can use objects for that, but it might be a little more complicated
var number = {
value:10
}
function test(number) {
number.value = number.value + 2;
}
test(number);
you might got an error here if number wont have any value, or you don't pass a number at all; so you should also use check
function test(number) {
if(number && number.value){
number.value = number.value + 2;
}
}
This will work, because when you pass an object (or array) you are passing a reference to that object, and it is possible to modify the contents of that object.
Hope that helps and wont confuse you.
Jason Desiderio
21,811 PointsJason Desiderio
21,811 PointsIt is something you'll have to pay attention to while you're writing the code. As you start writing larger applications, name spacing becomes a much much bigger concern. You will find quickly that writing a variable to the global name space is generally a bad idea and you should keep everything at the function level.