Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial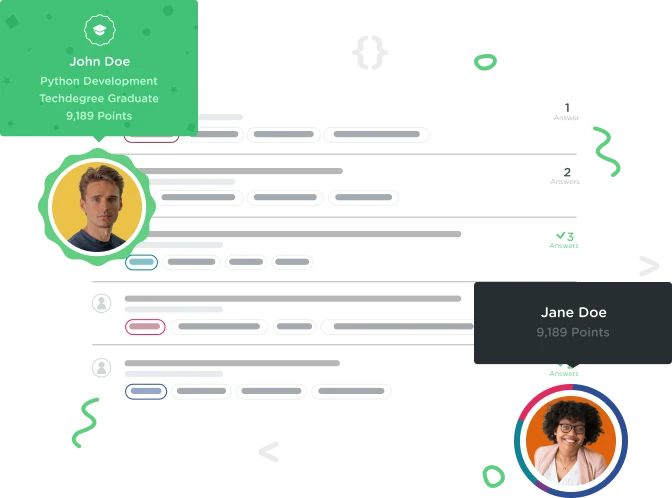
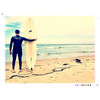
Samuel Mamulaschwili
9,935 Pointsfunction parameters
Hey, I am playing around with functions and need some help.
What I am trying to achieve: I want to use a function to grab the object properties and use them to generate my view. It works so far. Right now there a three functions (addRatingHtml, addRatingCSS, addRatingJS), they do nearly the same. I want to reduce those three functions, instead use only one function and pass some parameters in there. But I have no idea how to do this?"
My code so far:
//Skills Content
var HTMLSkillsItem = '<div class="col-md-4 skill-item"><p>%data%</p></div>';
var HTMLSkillsRating = '<span class="glyphicon glyphicon-star"></span>';
var skillsSet = {
"skill" : [
{
"skillType" : "Html",
"skillRate" : 4
},
{
"skillType" : "CSS",
"skillRate" : 4
},
{
"skillType" : "Javascript",
"skillRate" : 2
}
]
};
for(var skill in skillsSet.skill) {
var DYNHTMLSkillsItem = HTMLSkillsItem.replace('%data%', skillsSet.skill[skill].skillType);
$('.skills:last').append(DYNHTMLSkillsItem);
}
function addRatingHtml() {
for( var i=0; i < skillsSet.skill[0].skillRate; i++) {
$('.skill-item:first').append(HTMLSkillsRating);
}
};
function addRatingCSS() {
for( var i=0; i < skillsSet.skill[1].skillRate; i++) {
$('.skill-item:nth-child(2)').append(HTMLSkillsRating);
}
};
function addRatingJS() {
for( var i=0; i < skillsSet.skill[2].skillRate; i++) {
$('.skill-item:nth-child(3)').append(HTMLSkillsRating);
}
};
addRatingHtml();
addRatingCSS();
addRatingJS();
1 Answer
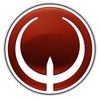
Leo Tank
Full Stack JavaScript Techdegree Student 36,270 Pointsfunction addRatingSkill(skillsSet, skill) {
var skillIndex;
switch (skill) {
case 'html':
skillIndex = 0;
break;
case 'css':
skillIndex = 1;
break;
case 'js':
skillIndex = 2;
break;
default:
return;
}
if (skillIndex) {
for (var i = 0; i < skillsSet.skill[skillIndex].skillRate; i++) {
$('.skill-item:nth-child(skillIndex)').append(HTMLSkillsRating);
}
}
}
rydavim
18,814 Pointsrydavim
18,814 PointsIt's a bit difficult to work with only the script when it is designed to interact with an HTML page. Could you provide your HTML and CSS code as well?
As far as general advice goes, you would want to compare your three functions and identify what is different between them. You would then need to create a general function and pass in the differences with one or more variables. In this case, you'll probably only need the index of the skill you're trying to append.
If you're able to post the rest of your code, I'd be happy to help and get back to you with some ideas.
Happy coding!