Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial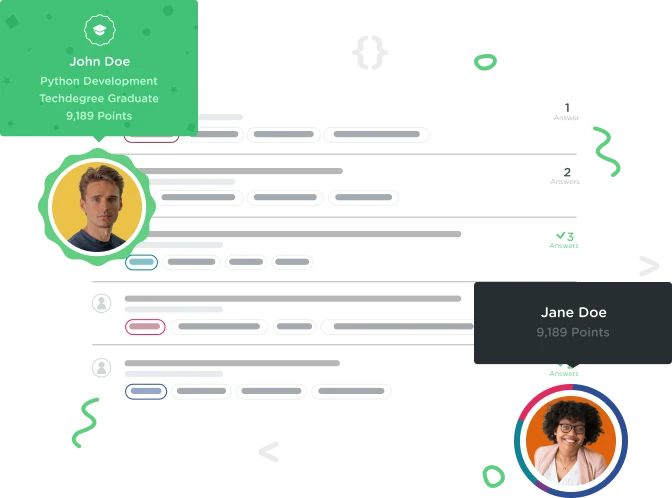

Anna Parker
Courses Plus Student 4,399 Pointsfunction return
I'm confused by this challenge, particularly this piece of code:
var year = new Date().getFullYear();
Specifically, I don't understand the var with two functions, or what the word 'new' means. Why isn't it connected to 'Date'?
Thanks!
function getYear() {
var year = new Date().getFullYear();
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
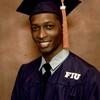
Dane Parchment
Treehouse Moderator 11,075 PointsI think you may need to re-watch a few videos to better understand objects and functions but I will walk you through understanding this so here we go:
So what is this new thing? So new basically creates a..well...new instance of an object that can be used or attached to a variable.
function Dog(name, species, noise) {
this.name = name;
this.species = species;
this.bark = function() {alert(noise)}
}
So if we look at the function above we see that we have a Dog function, that has the properties: name, species, and bark. Both name and species are relatively simple variable, but bark is a function. If we wanted to create a dog, we would need to assign a variable to that function. But there has to be a better way right? What if I want to have multiple dogs? Well that is where new comes in. New allows us to create a new instance of this dog function and assign it to something (or just access its properties. So let's do just that:
var lucky = new Dog("lucky", "dalmation", "woof");
var buster = new Dog("buster", "yorkie", "yip");
Now let's say that we just want to get the bark of a dog, we can do it like this:
var bark = new Dog("lucky", "dalmation", "woof").bark();
Calling bark would cause an alert with text "woof".
Are you getting it now?

Anna Parker
Courses Plus Student 4,399 PointsI rewatched the video, and I get your example (thanks!), but I don't understand how to return the variable from the function in the challenge question.
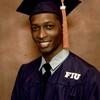
Dane Parchment
Treehouse Moderator 11,075 PointsOk, so basically you are creating a function called getYear():
function getYear() {}
Then we need to create a variable that retrieves the getFullYear of the Date object and return it:
function getYear() {
var year = new Date().getFullYear();
return year
}
Effectively this creates a variable that holds the value of getFullYear() and then makes the function return that value, so that whenever we call getYear() it returns the current value of the year variable. So the next step is calling the function to get said value:
function getYear() {
var year = new Date().getFullYear();
return year;
}
var yearToday = getYear();
And that's it you are done!

Anna Parker
Courses Plus Student 4,399 PointsThanks for your patience!