Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial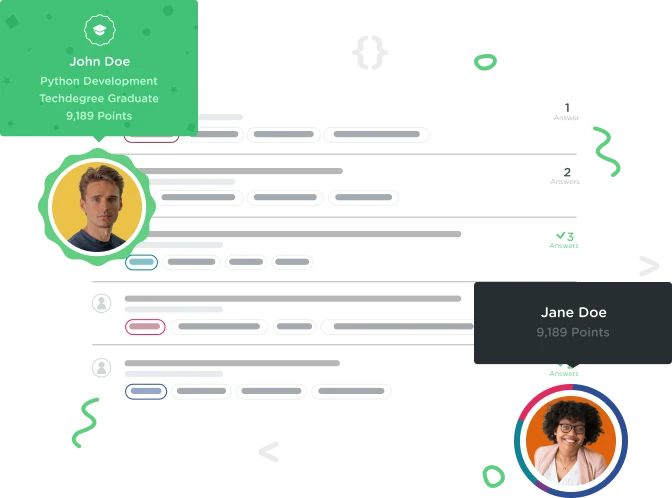

Ken Bynell
5,934 PointsFunction returns a combined datetime object, but I'm still getting the wrong answer.
This is the way I did it, unless maybe there's an alternate method using operators? Or maybe the solution is looking for something else? I even ran an isinstance test on combined_date and datetime came back true. Not really sure what else to do here.
from datetime import datetime
def time_tango(user_date, user_time):
combined_date = datetime.combine(user_date, user_time)
return combined_date
2 Answers
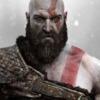
boi
14,242 PointsYou got two errors. Let us analyze.
from datetime import datetime π# what is the first datetime? you only need import datetime
def time_tango(user_date, user_time):
combined_date = datetime.combine(user_date, user_time)π# datetime.combine is a wrong package
return combined_date
datetime.combine()
is a wrong pacakage or datetime don't have the extension of combine()
, check it yourself.
try this in the REPL;
>>> import datetime
>>> dir(datetime)
['MAXYEAR', 'MINYEAR', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__'
, 'date', 'datetime', 'datetime_CAPI', 'sys', 'time', 'timedelta', 'timezone', 'tzinfo']
>>>
>>> dir(datetime.datetime)
['__add__', '__class__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__',
'__init__', '__init_subclass__', '__le__', '__lt__', '__ne__', '__new__', '__radd__', '__reduce__', '__reduce_ex__', '__repr__', '__rsub__
', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', 'astimezone',π 'combine' π, 'ctime', 'date', 'day', 'dst', 'fold',
'fromisocalendar', 'fromisoformat', 'fromordinal', 'fromtimestamp', 'hour', 'isocalendar', 'isoformat', 'isoweekday', 'max', 'microsecond'
, 'min', 'minute', 'month', 'now', 'replace', 'resolution', 'second', 'strftime', 'strptime', 'time', 'timestamp', 'timetuple', 'timetz',
'today', 'toordinal', 'tzinfo', 'tzname', 'utcfromtimestamp', 'utcnow', 'utcoffset', 'utctimetuple', 'weekday', 'year']
>>>
As shown, there is no combine()
extension with datetime
, it should be datetime.datetime.combine()
.
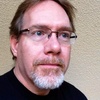
Chris Freeman
Treehouse Moderator 68,457 PointsI think thereβs more going on here. All of these should work equally as well:
# as module import
>>> import datetime
>>> help(datetime.datetime.combine)
Help on built-in function combine:
combine(...)
date, time -> datetime with same date and time fields
# as renamed class import
>>> from datetime import datetime as dt
>>> help(dt.combine)
Help on built-in function combine:
combine(...)
date, time -> datetime with same date and time fields
# as direct class import
>>> from datetime import datetime
>>> help(datetime.combine)
Help on built-in function combine:
combine(...)
date, time -> datetime with same date and time fields
This needs further review.
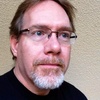
Chris Freeman
Treehouse Moderator 68,457 PointsUsing the REPL example:
>>> from datetime import datetime
>>> dir(datetime)
[β__add__β, β__class__β, β__delattr__β, β__dir__β, β__doc__β, β__eq__β, β__format__β, β__ge__β, β__getattribute__β, β__gt__β, β__hash__β, β__init__β, β__le__β, β__lt__β, β__ne__β, β__new__β, β__radd__β, β__reduce__β, β__reduce_ex__β, β__repr__β, β__rsub__β, β__setattr__β, β__sizeof__β, β__str__β, β__sub__β, β__subclasshook__β, βastimezoneβ, π'combine'π, βctimeβ, βdateβ, βdayβ, βdstβ, βfromordinalβ, βfromtimestampβ, βhourβ, βisocalendarβ, βisoformatβ, βisoweekdayβ, βmaxβ, βmicrosecondβ, βminβ, βminuteβ, βmonthβ, βnowβ, βreplaceβ, βresolutionβ, βsecondβ, βstrftimeβ, βstrptimeβ, βtimeβ, βtimestampβ, βtimetupleβ, βtimetzβ, βtodayβ, βtoordinalβ, βtzinfoβ, βtznameβ, βutcfromtimestampβ, βutcnowβ, βutcoffsetβ, βutctimetupleβ, βweekdayβ, βyearβ]
>>>

Ken Bynell
5,934 PointsSweet, thanks. I'm using my own IDE and the command prompt and have different imports for both, so it was probably getting confused.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsDevs: this code should pass. The level of import should not matter in this case. Please review checker behavior.