Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial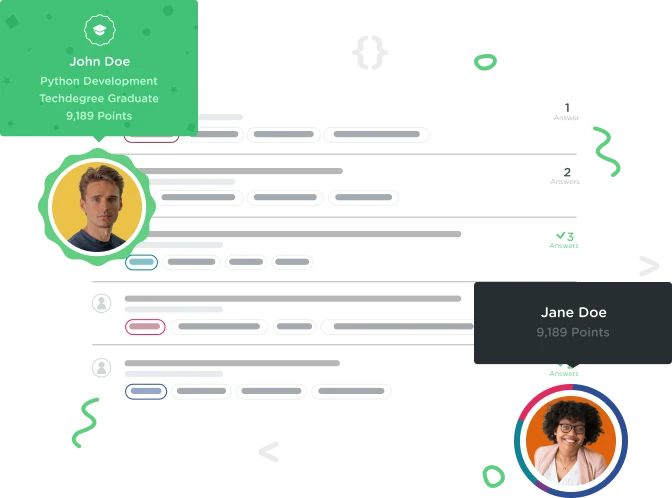

Maor Tzabari
1,762 PointsFunction that return the high number
Hey, I couldn't solve this question
My code is attached, I think i did a mess
function max( input1, input2, high ) {
var num1 = parseInt(input1);
var num2 = parseInt(input2);
if ( num1 > num2 ) {
var high = num1;
}
else {
var high = num2;
}
}
max(high);
4 Answers
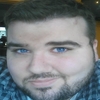
Marcus Parsons
15,719 PointsHey Maor Tzabari,
Although your function is syntactically correct, it is less efficient than the actual answer because it uses more memory and isn't returning anything. You only need two arguments: an input1 and input2. There's no need for a variable named high because you want to return the higher number.
For example, let's say I were making my own "min" function:
//Create the function called min with two arguments: an input1 and an input2
function min(input1,input2){
//If input1 is less than input2, it is the minimum so
if (input1 < input2) {
//Return input1
return input1;
}
//Otherwise, input2 must be smaller so
else {
//Return input2
return input2;
}
}
That example above is very similar to the way you should construct your max function. I don't want to give you the answer directly because I want you to see what is happening and figure it out for yourself. It is far more satisfying that way, as well. :)

Ben Singer
8,621 PointsYou've not passed any numeric arguments to the function.
That is to say: you've put no numbers in, so the function has nothing to compare against.
You've currently got :
max(high)
What you need is something like:
max(40, 76);
Also, there's no need to put high in the function arguments, just make it a variable and then return the value of that variable at the end of the function i.e.:
function max( input1, input2 ) {
var num1 = parseInt(input1),
num2 = parseInt(input2),
high;
if ( num1 > num2 ) {
high = num1;
}
else {
high = num2;
}
return high;
}
max(40,76);

Maor Tzabari
1,762 PointsI did it also before, but it didn't work

Ben Singer
8,621 PointsYou did what before?
Both the examples on the page work fine, I've tried them in codepen with no problems, but Marcus' is a better solution as it's more efficient.
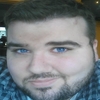
Marcus Parsons
15,719 PointsBen, you haven't taken into account that this is a challenge and that your code is convoluted and unnecessary. You are initializing a variable for no reason just to return that variable. It is much more efficient to just return which input was larger which is exactly what the challenge wants and is a best practice.

Ben Singer
8,621 PointsI hope you're as fun a guy off the forum as you are on it.
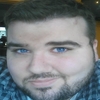
Marcus Parsons
15,719 PointsI must not have read your comment correctly. I didn't mean for that to come out harsh sounding. Apologies!

Ben Singer
8,621 PointsNo worries :)

Maor Tzabari
1,762 PointsWhat I'm saying, I did exactly like you efficient code before, and it still gave me error, that's why I changed my code, so you saw only this version of what i wrote. Anyway, I think I got it
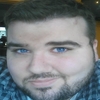
Marcus Parsons
15,719 PointsI didn't write out the answer for you. I wrote a similar example so that you could grasp what's going on because a basic min and a basic max function are almost the same in their execution. But the answer to the challenge is:
function max(input1,input2){
if (input1 > input2) {
return input1;
}
else {
return input2;
}
}