Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial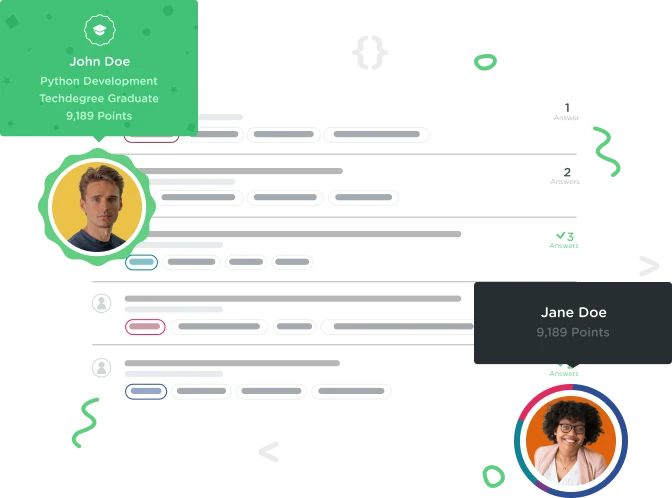
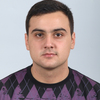
Georgi Koemdzhiev
12,610 PointsFunction that returns the word frequency in a string?
I am trying to solve a code challenge where I have to write a method that counts the words in a sentence. I have tested my code in pyCharm and it works. However, the online python interpreter does not want to accept my method as an answer to the challenge. Any ideas what I am missing?
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(myString):
output_dict = {}
for word in myString.lower():
current_letter_counter = 0
for word2 in myString:
if word == word2.lower():
current_letter_counter += 1
if word == ' ':
pass
output_dict.update({word : current_letter_counter})
return output_dict
1 Answer
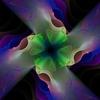
Travis Howk
7,169 PointsThe code that you are running has a very high iteration rate. Part of the problem is that your first for loop is iterating over every single letter in the string (not the words). As such, you might be better off splitting your strings using something like the .split() method, which would allow your string to be broken up by a certain character like a space. It would look something like this:
my_string = "This is my sentence. I hope my code works."
my_array = my_string.split(" ")
Then you would have my_array = [This, is my, sentence., I, hope, my, code, works.] That would make it a bit easier to deal with.
Edit: Here is my code
def word_count(myString):
output_dict = {}
myarray = myString.split()
for word in myarray:
if word.lower() not in output_dict:
output_dict[word.lower()] = 1
else:
output_dict[word.lower()] = output_dict[word.lower()] + 1
return output_dict
Georgi Koemdzhiev
12,610 PointsGeorgi Koemdzhiev
12,610 PointsThanks for the help, Travis!