Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial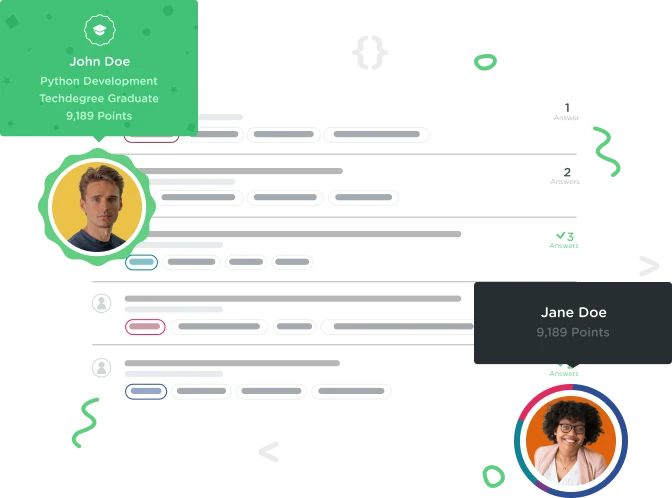

Jonathan Fernandez
7,542 PointsFunction works fine in console but acts weird when called upon
Hi everyone,
So I’m trying to figure out why this function works well in the console but not when called. I’ve tested my prompt variables on another simpler function area() and it seems to work fine. I even had the randomInBetween function return the var most and that checks out too! Something really funny happens once it gets to the var answer in the function I think. Since it’s working in the console, just what kind of data is being passed into the answer equation exactly???
Shown below is my code:
function randomInBetween(num1, num2) {
parseInt(num1, num2);
if (num1 > num2) {
var most = num1;
var least = num2;
} else if (num1 < num2) {
var most = num2;
var least = num1;
} else {
return num1;
}
var answer = Math.floor(Math.random() * (most - least + 1)) + least;
return answer;
}
//function area(width, legth) {
// var area = width * legth;
// return area;
//}
var globalNum1 = prompt("Enter Number 1");
var globalNum2 = prompt("Enter Number 2");
var globalAnswer = randomInBetween(globalNum1,globalNum2);
//var globalAnswer2 = area(globalNum1, globalNum2);
alert("Your random Number is " + globalAnswer);
//alert("Test " + globalAnswer2);
Any feedback would be deeply appreciated! : )
5 Answers

Jonathan Fernandez
7,542 PointsHi everyone,
After experimenting with the code I have finally found a solution as to why it works in the console but not the alert box. I had been trying to assign strings to the variable most and least, given that the input is provided in strings and not integers.
I assigned new variables (clean1 & clean2) to hold the parse-Int values and replaced num1 & num2 with these new variables all over the function to get a clean int calculated result.
Shown below is my code for the fix:
var most;
var least;
function randomInBetween(num1, num2) {
var clean1 = parseInt(num1);
var clean2 = parseInt(num2);
if (clean1 > clean2) {
most = clean1;
least = clean2;
} else if (clean1 < clean2) {
most = clean1;
least = clean2;
} else {
return clean1;
}
var answer = Math.floor(Math.random() * (most - least + 1)) + least;
return answer;
}
var globalNum1 = prompt("Enter Number 1");
var globalNum2 = prompt("Enter Number 2");
var globalAnswer = randomInBetween(globalNum1,globalNum2);
alert("Your random Number is " + globalAnswer);
Once again thank you all for your time with this. Meant a lot! : )

Ryan Zimmerman
3,854 Points1st I would declare your variables before the if statement but I am not sure what your goal is.
What are the difference in returns?

Jonathan Fernandez
7,542 PointsHi Ryan,
Thanks for your reply and advice on the variable declarations. Will keep this in mind for cleaner coding. : )
The goal for this is to have the user input 2 values and return a random value in-between. The if statements are there to distinguish the highest value and least value or check if they are equal. If they are equal it will just return num1 as it's random generated number.

Erik Nuber
20,629 PointsThere are a couple of problems in the function.
parseInt(num1, num2);
Here num1 is a string to be made into an integer, num2 is a radix value. So it is not doing what you think it is. You need to separate them
parseInt(num1);
parseInt(num2);
Next there is a problem with your random number generator it is missing a ")"
var answer = Math.floor(Math.random() * (most - least + 1) + least);
I tried both of these fixes and it worked for me just fine. I didn't test it to death but, tried several variations.

Jonathan Fernandez
7,542 PointsHi Erik,
Thanks for your reply and time for this.
Also thanks for pointing out the ")". I edited my post moments after posting and had added the extra ")". Although the original equation shown below is what should be followed with *least * variable being excluded from the ")".
Link: JavaScript Solution Video
var answer = Math.floor(Math.random() * (most - least + 1)) + least;
So after trying it with the fixed equation and separating parseInt for num1 and num2 I still can't get it to work. How do these work for you? Did you do an alert and console test with it? The results are extremely different for me. In the alert I sometimes even get negative values!! It's driving me crazy haha

Erik Nuber
20,629 PointsI used this site to test the code.
http://www.webtoolkitonline.com/javascript-tester.html
you just copy paste your code in and can execute it immediately with the push of a button.
I also was having some really strange results with your code. Even got a double zero back which was surprising.
The only other things I did were setting the most/least variable variables inside the function to ""
var most = ""
var least = ""
I did that because you get warnings that most and least are already defined. However, it doesn't appear to effect the code.
Try your code at that site. I just did it again and still there are no issues no matter how you put the numbers in.

Jonathan Fernandez
7,542 PointsHi Erik,
Thanks for the feedback. I have tried adding empty string values to the variables as you suggested but it still gave me weird behavior. Although I got it working and have shared my solution on this thread. : )

Erik Nuber
20,629 PointsDoesn't particularly matter in this case but I would add your variables into the function to make them local variables. As you have them they are global. Also the code still doesn't have the ")" after the final least. This then makes a random number gets the "floor" and then adds in the least.