Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial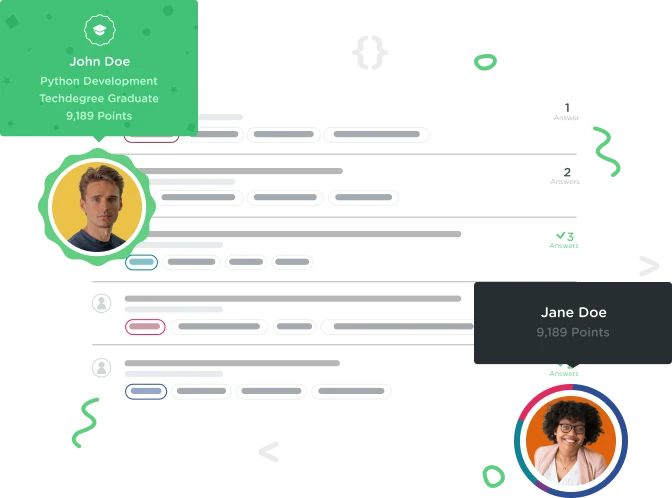
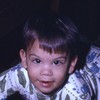
Adam Raitano
5,387 Pointsfunctional programming 'datetime' confusion
Hi,
So, I am working on step one of the "map/filter" code challenge in the functional programming lesson. It's been a while since I've dealt with the datetime stuff so I am going back and am doing some refreshers. This is all well and good.
The problem I am having is that the 'Bummer' commentary isn't that helpful when it comes to debugging a problem so what I often do is write the code in Idle or Notepad++ in order to get more practice coding as well as getting any pertinent error messages that might help me reach my goal.
Now, I have gone to my Idle editor and typed in exactly what the original code is (minus my function) and when I run it I get this error:
"line4, in <module> datetime.datetime(2014, 4, 29), TypeError: 'module' object is not callable."
Can someone explain what is happening?
Thanks
import datetime
birthdays = [
datetime.datetime(2012, 4, 29),
datetime.datetime(2006, 8, 9),
datetime.datetime(1978, 5, 16),
datetime.datetime(1981, 8, 15),
datetime.datetime(2001, 7, 4),
datetime.datetime(1999, 12, 30)
]
today = datetime.datetime.today()
def is_over_13(td):
return today.days - td.days >= 4745
5 Answers
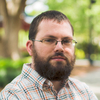
Kenneth Love
Treehouse Guest Teacherdef is_over_13(today,dt):
how_many_days = today - dt
return how_many_days.days >= 4745
Your function doesn't need to accept today
, though. today
comes from the global scope. Other than that, your code looks correct to me.
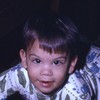
Adam Raitano
5,387 PointsThat error doesn't make sense unless you named your file datetime.py or something like that.
bingo. Thanks.
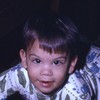
Adam Raitano
5,387 PointsAs long as I have your attention. I've been working on this for a bit and I think I don't fully comprehend what it is you are asking for. the function takes a datetime but needs to determine the difference between two. Here is my attempt
def is_over_13(today, dt):
return today.days - dt.days >= 4745
This is clearly not correct. Any advice?
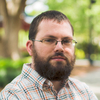
Kenneth Love
Treehouse Guest Teacherdatetime
objects don't have a days
attribute. timedelta
objects do, though. You need to create the timedelta
and then compare the days
attribute to the target.
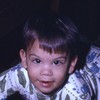
Adam Raitano
5,387 PointsHmmmm.....
I tried this
def is_over_13(today,dt):
how_many_days = today - dt
return how_many_days.days >= 4745
to no avail.
(insert interrobang here)
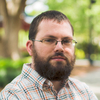
Kenneth Love
Treehouse Guest TeacherThat error doesn't make sense unless you named your file datetime.py
or something like that. Importing datetime
and calling datetime.datetime()
is 100% valid.
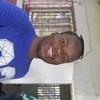
Innocent Ngwaru
7,554 Pointsi passed with this function after a bit of a struggle
def is_over_13(dt): """Is the difference between datetime and today 4745 days or more""" difference = today - dt return difference.days >= 4745
Adam Raitano
5,387 PointsAdam Raitano
5,387 PointsI see. Thanks, that worked. I guess my understanding of scope in this context is lacking...
Ethan Chae
18,747 PointsEthan Chae
18,747 PointsDear Kenneth, I have similar code to yours but it doesn't pass.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherEthan Chae Yeah, read the comment. That code is Adam's and it's wrong. The wrongness is explained in the comment.