Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial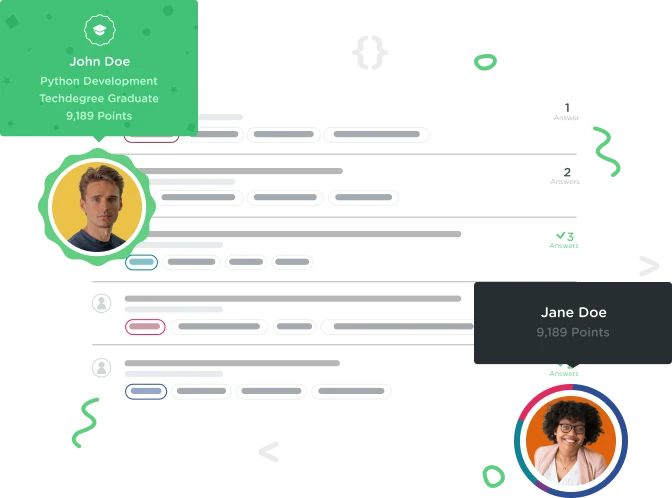

rymatech
4,671 PointsFunctional programming JavaScript: best way to incorporate an array into functions?
I am building a calculator and I have the below array outside of the function and then the function mutates it, which I understand is poor coding practise (not functional).
How would I incorporate the array into the function, keep in mind there will be other functions (plus, minus etc) which also need to access the array and updated data within it.
let arr = []; // Store user input.
let str = ''; // Display user input in the display section.
decimal.addEventListener('click', () => {
arr.push('.');
str = str.concat('.');
display.value = str;
});
plus.addEventListener('click', () => {
arr.push('+');
str = str.concat('+');
display.value = str;
});
There are more siminlar functions such as plus, 2, 1, 3, 4, 5, 6 etc.
4 Answers
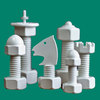
Steven Parker
231,275 PointsConsidering what you use the array for, and that it is a global element, I don't see any issue with the usage shown here. What led you to think it was bad practice? (Maybe I've been doing things wrong myself!)
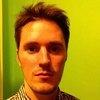
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI haven't run your code, so sorry if this is a drive-by opinion. But I noticed that you're using jshint, but you have some issues that jshint is complainng about that are not addressed. Specifically:
-
eval
can lead to security issues (this security course will explain why) - declaring functions within a loop using the loop variable can lead to confusion and bugs. And there's usually a better way: I'm not sure why it's necessary to loop through all the operators in one big function, and assign different behavior to them depending on the operator. Seems to me each operator could just have it's own event handler that takes care of it's needs.

rymatech
4,671 Points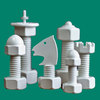
Steven Parker
231,275 PointsArray indices go up to but not including the length of the array:
for (let i = 0; i <= numButtons.length; i++) { // change "<=" to just "<"

rymatech
4,671 PointsSteven Parker Perfect thanks for the help.
Do you think my logic is okay?
I am trying to program more functionally.
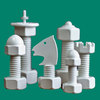
Steven Parker
231,275 PointsLooks good to me. Considering what the page does, I think the use of global storage is appropriate, but it shouldn't be too hard to encapsulate the whole thing if you really want to.
rymatech
4,671 Pointsrymatech
4,671 PointsI've just started learning about functional programming and perhaps i've misunderstood it.
I thought that the idea is to have everything within functions in order to reduce bugs, make it more testable and modular. Also important not to mutate anything in the global scope.
This then made me think perhaps I should be using closures to incorporate the array into a function?
Below is my current JS calculator code which I am planning to rewrite. The below code also fails in to console. with the following message:
"Uncaught TypeError: Cannot read property 'addEventListener' of undefined at loopNumberButtons (scripts.js:47) at scripts.js:136"
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIf your intent is to create a module and not a whole program, then yes, encapsulation is a good idea. But closures are generally not necessary any more if you make use of the modern (ES2015) syntax extensions.
If you share the HTML portion of the code also, I'll see if I can spot the issue. Even better, make a snapshot of your workspace and post the link to it here.
In the meantime, I spotted this: