Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial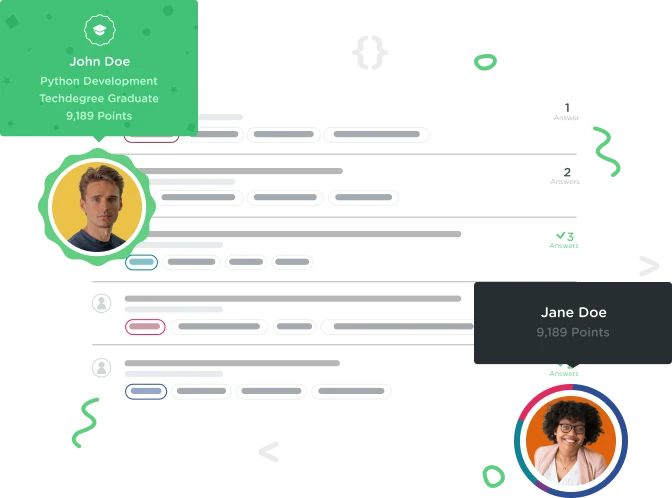

orange sky
Front End Web Development Techdegree Student 4,945 Points(function(){...})(arg1, arg2)
Hello!
In the lecture video, the instructor shows us how to create a function with a private scope, however he doesn't show how to pass arguments into it. Can someone please show me a small example that takes in arguments and output something using this function style:
(function () {
var a, b, c;
//....
})(arg1, arg2)
thanks!
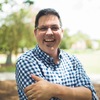
Dave McFarland
Treehouse TeacherTo put code into a forum post use triple back ticks -- ``` — around the code. I fixed your code here, but in the future here's a forum discussion that describes how to add HTML, CSS, JavaScript or other code to the forum: https://teamtreehouse.com/forum/posting-code-to-the-forum
4 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsAn immediately-invoked function expression (IIFE) can be named, but that's usually only useful for debugging purposes (or recursive functions):
(function myIIFE() {
// some code
})();
And yes, you can pass parameters to it, like this:
var color = "red";
var otherColor = "purple";
(function (favColor, anotherColor) { // the IIFE accepts parameters favColor and anotherColor
var statement = "My favorite color is ";
console.log(statement + favColor); // the first parameter is logged here
console.log("I also like " + anotherColor); // the second parameter is logged here
})(color, otherColor); // passing color and otherColor as the parameters for the IIFE

orange sky
Front End Web Development Techdegree Student 4,945 PointsFrom your example, it does not seem too hard, but I think I should wait to learn this concept

orange sky
Front End Web Development Techdegree Student 4,945 PointsI have seen the word recursive a few times; now I see it means debugging. Is this (recursive) a topic I will learn in another Javascript course. I should not try to understand it now, right? Thanks!!!

Dino Paškvan
Courses Plus Student 44,108 PointsActually recursive does not mean debugging. I was listing an additional example. Recursive functions are functions that call themselves:
function myFunc() {
// some code
myFunc();
}
Recursion can be really complex, so you probably shouldn't worry too much about it right now.

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Dave, I thought it was my imagination; I was starting to wonder why I can post a full length code when I start a brand new discussion, but when I respond, the code is never formatted. Thank you for the heads up!!!!

orange sky
Front End Web Development Techdegree Student 4,945 PointsGot it!! I will keep taking my baby steps, and worry about it then. Thanks Dino!
orange sky
Front End Web Development Techdegree Student 4,945 Pointsorange sky
Front End Web Development Techdegree Student 4,945 PointsAlso, I wonder if this kind of function ( (function () { var a, b, c; //.... })(arg1, arg2) can have a name like: var myFunction = function(){.....}, or function myFuncntion(){ ...}
thanks!!