Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial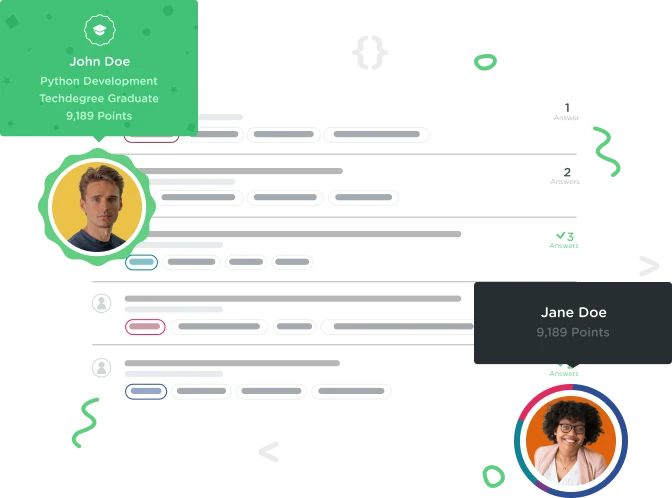

Akhilesh Bhartiya
Courses Plus Student 355 Pointsfunctions
include <stdio.h>
float addTwo (float result);
int main ()
{
addTwo(result);
return 0;
}
float addTwo (float result) {
int a = 10;
int b = 20;
result = a + b;
printf("%0.1f\n", result);
return result;
}
why can't we do like this ?? why do we have to create another float in main to make it run ?
3 Answers

eirikvaa
18,015 PointsThe challenge description will tell you that you don't have to write the main function, just the function implementation.
There are a couple of things you should note about your function.
- You don't need the result variable. Just use the two variables.
- You don't have to print the result. Just return it.
Hope this helps.
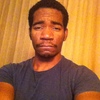
Stephen Whitfield
16,771 PointsBecause the argument you're passing, you're setting it to something else in the function and returning it. If you want your function to work, pass it arguments that are added and return the result. For example:
float addTwo(float a, float b) {
float result = a+b;
return result;
}
//And in main():
addTwo(16.3, 5.2); //returns 21.5

Akhilesh Bhartiya
Courses Plus Student 355 Pointsyou not understanding the question its not a point of using different parameter, it doesn't effect if i use float return or float a, float b. its also not about inside the addTwo function. its about calling a function, when i am calling my function addTwo why can't I call it like :- in main {addTwo (result) ;}, why do I have you create another floating variable and inserted it into addTwo function parameter.

Akhilesh Bhartiya
Courses Plus Student 355 PointsDo you want me to show you how this program is gonna run
float addTwo (float result);
int main ()
{
float c = 2;
addTwo(c);
return 0; }
float addTwo (float result)
{
int a = 10; int b = 20; result = a + b;
printf("%0.1f\n", result);
return result;
}
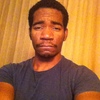
Stephen Whitfield
16,771 PointsYou don't have to create a typedef float as an argument to your function, so as long as you are returning a typedef float result. Do you understand? You could just as well using an integer as your parameter, but you can't expect the integer to be returned as an integer if the typedef of your function is a float. I hope that isn't confusing.

Akhilesh Bhartiya
Courses Plus Student 355 Pointsyou can use integer value in double but it doesn't work other way around, but thats not the answer of my question
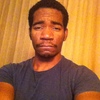
Stephen Whitfield
16,771 Points^ You can if you convert the double to an int using (int)doubleValue. Anyways, I don't think I'll ever be able to answer your question without further insight as to what you're needing to know. Maybe someone else can be of help. Stone Preston Ben Jakuben Amit Bijlani

Akhilesh Bhartiya
Courses Plus Student 355 Pointshow can i contact to them

eirikvaa
18,015 PointsI understand your question. In your first example, inside the main function, you do like this:
addTwo(result);
But the compiler doesn't know anything about any result variable. Yes, you use the result variable inside the function implementation, but that's just a local variable. The main function doesn't know anything about it. So when you do like this:
float c;
addTwo(c);
Now the compiler has a variable to use. The variable c gets used in the function call and addTwo returns the correct value.
Akhilesh Bhartiya
Courses Plus Student 355 PointsAkhilesh Bhartiya
Courses Plus Student 355 Pointsi know this example is from the challenge question. I extended the program myself and came across this problem why do I have to create a float to run my function. how can i do it without creating a float. i can tell you how this program gonna run but i want to know why it doesn't work like i mentioned above.
float addTwo (float result);
int main ()
{
float c = 2;
addTwo(c);
return 0; }
float addTwo (float result)
{
int a = 10; int b = 20; result = a + b;
printf("%0.1f\n", result);
return result;
}