Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial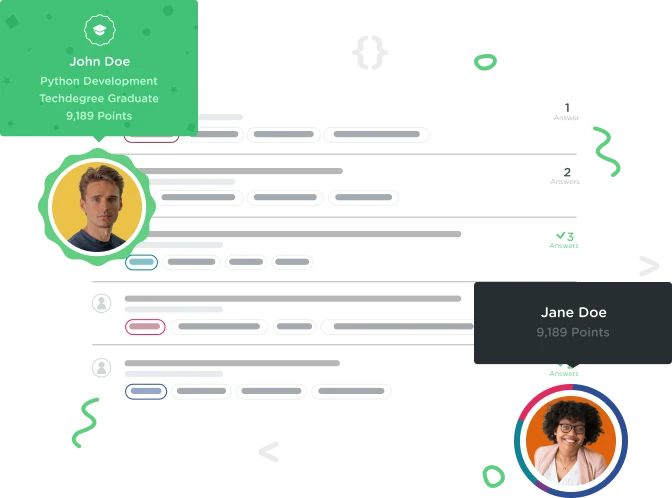
1 Answer

Thomas Nilsen
14,957 PointsI would do something like this:
(I added some comments. Let me know if something is still unclear. )
var list = [{id: "102", name: "Alice"}, {id: "205", name: "Bob", title: "Dr."}, {id: "592", name: "Clyde", age: 32}];
function groupBy(arr, callback) {
//Object that will contain the key/val pairs
var result = {};
//Loop through the arr we passed in
arr.forEach(obj => {
//When we execute our callback function
//We get back the value we want to groupBy
var key = callback(obj);
//If the key does NOT exist in the object,
//then create it and set it equal an empty array
if(!result.hasOwnProperty(key)) {
result[key] = [];
}
//Push the object that corresponds to our key
result[key].push(obj);
});
//We have our answer.
return result;
}
console.log(groupBy(list, function(obj) {
return obj.id;
}));
/*
This prints:
{ '102': [ { id: '102', name: 'Alice' } ],
'205': [ { id: '205', name: 'Bob', title: 'Dr.' } ],
'592': [ { id: '592', name: 'Clyde', age: 32 } ] }
*/