Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial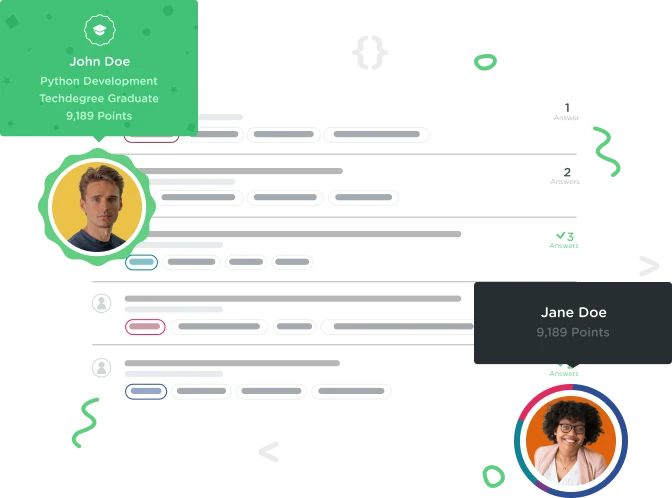

Sean Richardson
495 Pointsfunctions
I'm trying to understand the role of the () in def my_function (): and how its used and when. My understanding so far is that its an argument and that's about it. If for example the function is def my_function (item): does that mean I have to declare what item is ie item = ['cat', 'dog'] to use in the function. And what about when nothing is shown in () in the def statement, how and when is that used. I can't seem to find much in treehouse.
3 Answers

andren
28,558 PointsActually when defining a function the thing within the parenthesis is called a parameter, not an argument. Though arguments are related to this topic.
When you specify a parameter for a function you are stating that when the function gets called it needs to have a value passed in to it. And that said value will be assigned to that name within the function. That value by the way is what is referred to as an argument.
Take this example:
def my_function(word): # my_function takes has one parameter called word
print(word) # Print the contents of the word parameter
my_function("Hello, World!") # I'm calling my_function with one argument which is "Hello, World!"
The above code will result in "Hello, World!" being printed out. Specifying a parameter for your function makes it possible to pass data into the function when it is called, as demonstrated above. This is useful since it makes functions far more dynamic since they won't always work on the same data each time they are called.
Though it's worth noting that when you specify that your function requires a parameter as I show above then an argument has to be supplied when the function is called, not supplying an argument will lead to an error.

Sean Richardson
495 PointsThanks andren

Sean Richardson
495 PointsOnce again thanks Andren, its clearer now
Sean Richardson
495 PointsSean Richardson
495 PointsDoes your code set word to equal "Hello, World!" in the code or are they 2 separate examples?
andren
28,558 Pointsandren
28,558 PointsThey are not separate examples.
word
gets set to "Hello, World!" automatically, because a parameter is basically just a variable which is automatically set equal to whatever argument was passed in to the function.The first argument to the function is "Hello, World!" and the first parameter is
word
, thusword
is set to "Hello, World!".Here is another example:
In this example the first parameter
num1
is set equal to the first argument 5, and the second parameternum2
is set equal to the second argument 10. That is how parameter to argument pairing works. It is based entirely on their position, and separate parameters/arguments are separated by a comma.It is also worth nothing that an argument can be any type of value. I have demonstrated with Strings and numbers, but I could also have passed the contents of a variable or any other type of data you can think of.