Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial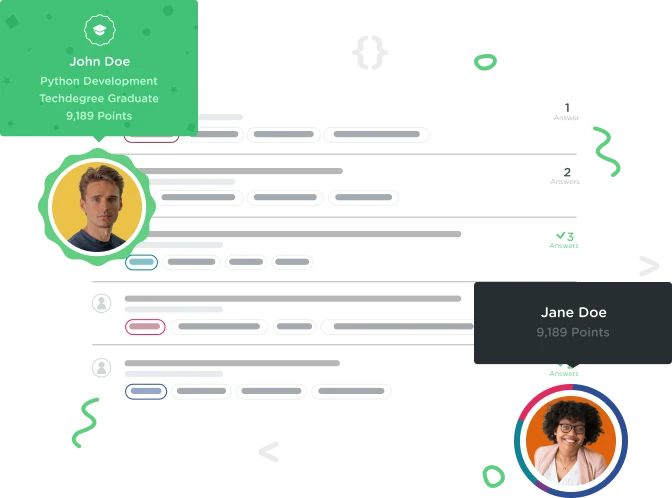
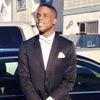
Reggie Sibley
6,018 PointsFunctions and Arguments
I just completed the Javascript basics tutorial, and i was stuck on Arguments for the longest time. i was able to complete the test with a simple understanding but i still cant wrap my head around how a FUNCTION and an argument should really work. I wanted to know if there where any additional tips/ resources someone could give me to understand really how arguments work. Anything will help! (I've already tried MDN and still having a hard time grasping the concept of arguments?) Mabey it requires alot of practice? but this is supposed to be the basics of javascript lol so i want a solid understanding before i move on to harder things.
5 Answers

Aaron Martone
3,290 PointsThe reason why we have functions is because they encapsulate a series of statements and can perform a task based on dynamic argument values passed to them. If you wrote: var a = 4 + 6;
, then a
is forever statically bound to the value 10
. And what good is that? I can't ever have a
equal anything else unless I procedurally reassign it a new value. Instead, if I have a function like:
function adder(x, y) { return x + y; }
Then I can call adder()
wherever I want (as long as its in scope) and then pass it dynamic values to have an operation done on them (in this case, adding two values and returning the result. This is infinitely more useful that simple static assignment.
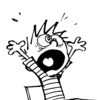
stevenstabile
9,763 PointsHi Reggie,
This is a good page to learn about JS functions
Arguments are the real values you pass into a function when calling it. They replace the parameters in the function declaration. For example, here we declare a function called add with 2 parameters, a and b. We're saying that when the add function is called, it should expect to be passed 2 arguments that it will add together and return the sum:
function add(a, b){
return a + b;
}
So if you wanted to add 5 and 3 together, they would be your arguments. You replace a and b with 5 and 3 when calling the function:
add(5, 3);
The function would look like this:
function add(5, 3){
return 5 + 3;
}
I hope that helps. Everything will take a fair amount of practice to get the hang of but JavaScript is a great language. Enjoy the journey!
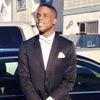
Reggie Sibley
6,018 PointsAppreciate the feedback, i understand the concept abit better now! But now my question is, why would i need a function to run code like that in particular when i can just create a variable? for instance...
var a = 2
var b = 2
var sum = a + b;
is it so that when i put all of this in a function, it performs all of these task? I think i answered my own question while coming up with this example, lol but i just want to be sure! And is it always necessary to return a value to a function?
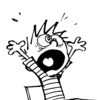
stevenstabile
9,763 PointsThere are a number of reasons why you should use a function for something like this but here are a couple of the main reasons:
DRY (Don't Repeat Yourself) - you should never repeat the same piece of code if it can be avoided. The example add function is very simple and we're only calling it once but what if you had 50 sets of numbers to add together? You'd have to set variables a and b to new values and then do something with the new sum value 50 times which is wildly inefficient, tedious, and it's very easy to make mistakes. The function can be called any number of times to do the work for you. In addition, you can pass the result from one function to another and so on.
Scope - Variables declared inside a function only exist within that function. This allows us to avoid conflicts that arise from declaring multiple global variables with the same name, or accidentally changing the value of a variable in one place that breaks your code in another place. In the following functions a and b basically act as placeholders for the arguments passed to them when each function is called. They don't exist outside of the functions and the a and b in one function has no relation to the a and b in any other function.
function add(a, b){
return a + b;
}
function subtract(a, b){
return a - b;
}
function multiply(a, b){
return a * b;
}
function divide(a, b){
return a / b;
}
Often times you will return a value in a function but you certainly don't have to. For example, the following function doesn't return anything:
function greeting(name){
alert("Hello " + name + ", it's nice to meet you!";
}
greeting("Reggie");
This will cause an alert box to pop up that reads: "Hello Reggie, it's nice to meet you!"
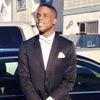
Reggie Sibley
6,018 PointsThanks so much guys for the help! i have a clear understanding of functions now! and much more research to do :)