Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial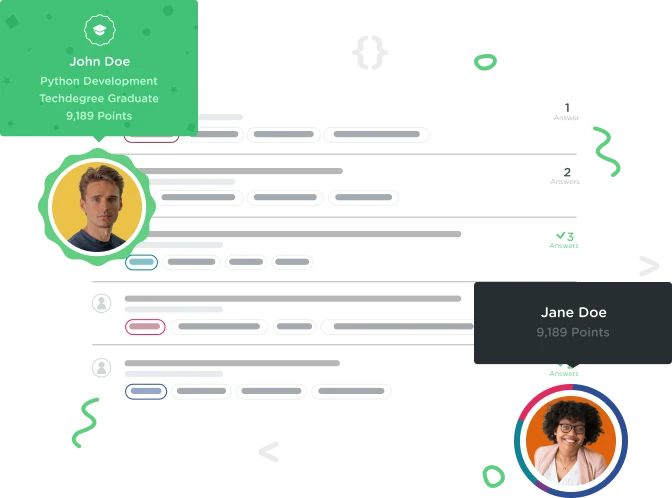

Christian Addison
1,945 Pointsfunctions are definitely not my strong suit so far... why won't it let me return these two?
It took me an hour to figure out it wasn't working because i didn't have a closing bracket at the end, and now it won't return the largest. I have the 2 variables be extremely different to test it out, but I'm lost.
function max(random, math) {
var random = Math.floor ( Math.random() * 10000) + 1;
var math = Math.floor ( Math.random() * 1) + 1;
if (random > math) {
return random;
} else if (random < math) {
return math;
} else {
alert('Error');
}
}
4 Answers
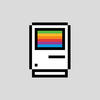
Hakim Rachidi
38,490 PointsCreate a max function
The challenge is to create a function which accepts to arguments and return the greater one so ...
- you don't need a random Number
- you don't need to override the parameter
you don't need to return something if they are equal (no else, no error alert)
you need a conditional structure to determine which value is greater
Hope this helps
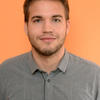
Adam Beer
11,314 PointsHi Christian! This is a simple task and your solution is too complicated. Use if/else statement inside the max functions. In the end use alert dialog with max function.

Edwin Rivera
Data Analysis Techdegree Student 4,895 PointsHey Christian Ill take a crack at this. so from your example you put up, i see you are trying to call the function while passing math and random which the variables do not exist outside the scope of the function. Also the else if statement for (random < math) seems a little unneeded because random will never be less than math.
I took your code and cleaned it up a little bit
function max() {
var math = Math.floor ( Math.random() * 1) + 1;
var random = Math.floor ( Math.random() * 10000) + 1;
if (random > math) {
return console.log(random);
} else {
return console.log(math);
}
}
max();
This way all you have to do is call max() and ever time it will call the variables inside the scope of the function and run the if statement and return your answer in the log menu.
i looked at the challenge you are trying to do and i see what you are trying to do. best example of tackling this would be to have the math and random, outside the function to pass into it.
var math = Math.floor ( Math.random() * 1) + 1;
var random = Math.floor ( Math.random() * 10000) + 1;
function max(math, random) {
if (random > math) {
return console.log(random);
} else {
return console.log(math);
}
}
max(math, random);
i hope this was able to help you :D first time answering a question
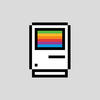
Hakim Rachidi
38,490 PointsHi Edwin,
This is a nice improvement to Christians current max function but I think Christian wants to accept the coding challenge. To do so he need to build a method which determines which value is greater

Matthew Lestina
Courses Plus Student 122 PointsThis is really confusing. To eliminate confusion, I would use differing variable names.
My 2 cents as an intermediate is to code:
var firstNum = Math.floor ( Math.random() * 1) + 1;
var secondNum = Math.floor ( Math.random() * 10000) + 1;
function max(math, random) {
if (random > math) {
return random;
}
else {
return math;
}
}
console.log(max(firstNum, secondNum));
Experts, please criticize if I am wrong.