Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial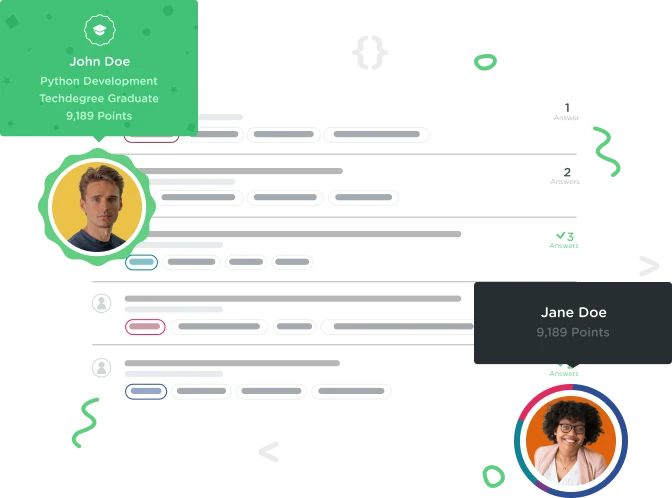
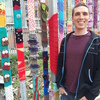
Brian Gallagher
9,074 PointsFunctions, Arrays and Return Values. Task 5
Very Stuck on this task. Can anyway offer the correct code?
Around line 17 create a function named arrayCounter that takes in a parameter which is an array. The function must return the length a=of an array passed in or '0' if a 'string', 'number' or 'undefined' value is passed in.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter (name, greeting, place) {
if (typeof name === 'undefined'); {
name = 0;
}
console.log(arrayCounter.length(name, greeting, place));
}
</script>
</body>
</html>
Thanks
3 Answers
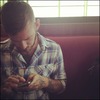
Erik McClintock
45,783 PointsBrian,
Let's break this down step by step.
1) They want you to declare a function called 'arrayCounter'. By now, we should know how to do this:
function arrayCounter() {
}
2) Make it so the function accepts one parameter, which will be an array. You can name this parameter anything you want; for clarity's sake, let's call it 'arrayParam':
function arrayCounter(arrayParam) {
}
3) Inside your function, you're going to return some value based on what the passed in argument is. Thus, we know we'll want to set up an 'if' statement:
function arrayCounter(arrayParam) {
if() {
//if return value here
} else {
//else return value here
}
}
4) The condition we want to check for is the type of the parameter passed in. If you'll recall from the lesson, there is a handy feature for this built into JavaScript called 'typeof':
function arrayCounter(arrayParam) {
if(typeof arrayParam) {
//if return value here
} else {
//else return value here
}
}
5) To make this simple, we can use a negative conditional to check if the value of our parameter is not what we're looking for. We should also know that an array is really just a list-like object, so we'll check if our parameter is not an object, and then return the corresponding value:
function arrayCounter(arrayParam) {
if(typeof arrayParam !== 'object') {
//we'll get here if the type of arrayParam is NOT an object, and thus NOT an array
return 0;
} else {
//else return value here
}
}
6) And so, if the type of arrayParam IS indeed an object, and thus IS an array, we want to return the length of that array, which we also recall from our previous lessons:
function arrayCounter(arrayParam) {
if(typeof arrayParam !== 'object') {
//we'll get here if the type of arrayParam is NOT an object, and thus NOT an array
return 0;
} else {
//we'll get here if the type of arrayParam IS an object, and thus IS an array, and since it IS an array, we can use array properties
return arrayParam.length;
}
}
Hope this has helped to break things down and show you the thought process that goes into putting a function like this together!
Erik

Aaron Graham
18,033 PointsThis seems to be a tough one for many, as there are several threads about this question. Try reading through this and see if it answers your question: https://teamtreehouse.com/forum/kinda-stuck-at-the-code-challenge-what-am-i-getting-wrong
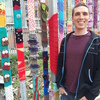
Brian Gallagher
9,074 PointsThanks a million Erik and Aaron, Fantastic explanation and really useful thread.
Brian
Aaron Graham
18,033 PointsAaron Graham
18,033 PointsReally well laid out, Erik McClintock.
thomas bethell
7,772 Pointsthomas bethell
7,772 PointsEric Awesome explanation. This one was hard.
Thanks!