Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial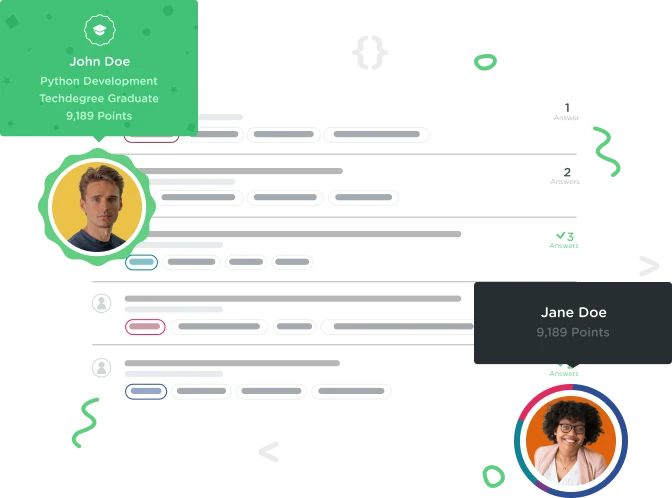

Peaches Stubbs
21,320 PointsFunctions code challenge
Hi the question that I'm stuck on is : Write a function named "addTwo" that accepts two float numbers as arguments. The function should also return a float which is the sum of the two arguments passed to the function.
I came up with:
int addTwo(int a, int b);
int main()
{
int a = 36;
int b = 37;
printf("add Two %d\n", addTwo(a, b));
return 0;
}
int addTwo(int a, int b);
{
return a + b;
}
The error message that I am receiving is : Bummer! Make sure you've defined the return type and both parameter types as 'float' and that you are returning the result.
May someone please point me in the right direction as ? Thanks !
13 Answers

eirikvaa
18,015 PointsThis is the full code for the solution and it is working for me. Take a look at it when you have the time.
float addTwo(float a, float b);
int main() {
float a = 2;
float b = 3;
printf("add Two %f\n", addTwo(a, b));
return 0;
}
float addTwo(float a, float b) {
return a + b;
}
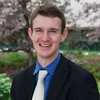
Spencer Sheehan
288 PointsI was having the same problem as you. What isn't made very clear in this problem (to a beginner) is that you don't have to declare the addTwo function and then main(), since that is technically already set up when you open a C program (but not really). I believe that is inferred when you are doing a challenge. What the question asks for is to just implement the function itself (which again, wasn't super clear to me). The challenge accepted this answer.
float addTwo(float a, float b) {
return a + b;}
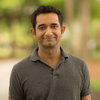
Amit Bijlani
Treehouse Guest TeacherYour answer is almost right. Firstly, the challenge is asking you accept and return a float
. So wherever you have an int
you should replace that with a float
. Next, all you need is the implementation of the function, which means just the last 4 lines from what you pasted above.

eirikvaa
18,015 PointsMake sure that your arguments and return type for the function addTwo are correct. You are using int, but they should all be floats. Also, in your format string you should use %f (floats) and not %d (integers).

Peaches Stubbs
21,320 PointsThank you both for your help. I changed the code to:float addTwo(float a, float b); float main() {
float a = 36; float b = 37;
printf("add Two %f\n", addTwo(a, b)); return 0; } float addTwo(float a, float b); { return a + b; }
Now its giving me this answer: Bummer! Make sure you've defined the return type and both paramter types as 'float' and that you are returning the result.
I honestly don't know what else I'm missing i did try changing the first return to a + b. Just to see if that would make a difference which it didn't. Went back to the answer above yet, still no dice.

eirikvaa
18,015 PointsLook closely:
float addTwo(float a, float b); {
There should not be a semicolon before the curly bracket. Remove it, and you're fine.

Peaches Stubbs
21,320 PointsThank guys, Eirik Vale AAse I tried what you said and it still isn't working. Maybe i've been fighting this too long. I'll take a break then watch the video and try again. Once again thank you :)
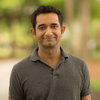
Amit Bijlani
Treehouse Guest TeacherSorry if you are having a hard time. But the complete answer to the code challenge is:
float addTwo(float a, float b) {
return a + b;
}
That's all you need.

Alexander Haggerty
1,850 PointsThanks, I was really struggling with this one.

Peaches Stubbs
21,320 PointsThanks for all your help guys, and it's okay that it's challenging that's what keep it interesting. :D

Peaches Stubbs
21,320 Points:D yes it did I passed it already but thank you .
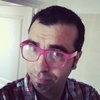
Francis Chouquet
290 PointsLike some people here I did declare everything. Actually my code was right but not accepted. I tried and tried again and then finally found the solution here :) I think the description is clear but I thought I had to do the whole stuff just like in the videos :P
Chris Howie
2,927 PointsI am trying to figure out where in the video training it relates these? I am just confused on how you came to this conclusion to start with. I am assuming as a new beginner/newbie that it is looking for just the last line of code? For example from the video
int funky_math(int a, int b) { return a + b + 343;
change all the int to floats?

Anthony Brown
5,175 PointsI think the main issue with this code challenge is that it is asking you to use floats. Floats are not mentioned until the next video after this particular code challenge.

Marcus Tegtmeier
1,852 PointsI was having the same problem as Spencer Sheehan. I think the question needs to be reworded so it's easier for beginners to understand.