Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial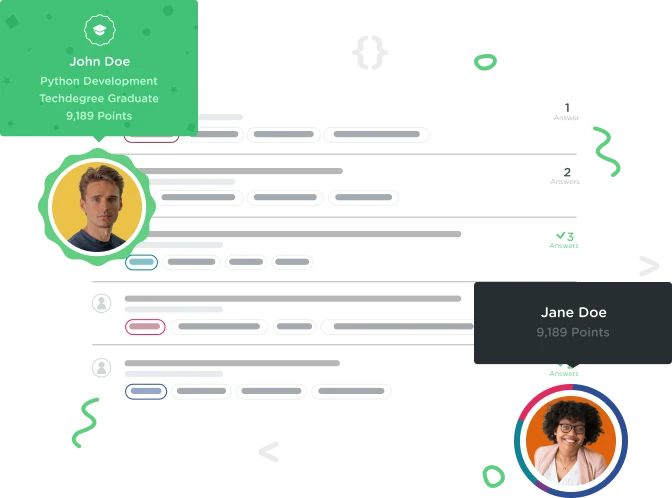

kj2
5,933 PointsFunctions Javascript
I may be thinking too hard about this, but I would like someone to explain in laman's terms as much as possible please, when you create a function in JS, when and when shouldn't you fill the parameters?
for example, I sometimes see
var exampleOne = function() {
// code here
}
and I see also
var exampleTwo = function (name) {
// code here
}
i just cant seem to wrap my head around the parameters part, any help would be great, thank you
3 Answers
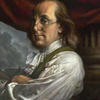
Jeff Busch
19,287 Points/*** The first example is a function that is
* expecting an argument (parameter). When the function
* is called in the first console.log, the number 5
* is passed to the function and the function returns
* the result of 5 * 5.
***/
var squareNummber = function($param) {
return $param * $param;
}
$numPassedTosquareNumber = 5;
console.log(squareNummber($numPassedTosquareNumber));
/*** In this example nothing is passed to the function
* when it is called. The function is called, does it's
* thing and returns a value.
***/
var squareNummber = function() {
var $numToSquare = 5;
return $numToSquare * $numToSquare;
}
console.log(squareNummber());
/*** This next example is really the same as the second
* one, it just "prints" the result from within the function.
***/
var squareNummber = function() {
var $numToSquare = 5;
consol.log($numToSquare * $numToSquare);
}
squareNumber();
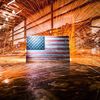
Andrew Shook
31,709 PointsI think the easiest way to explain it is that some functions preform tasks and some functions preform tasks on data. If your function preforms a task on specific data then you pass that data into a function as an argument/parameter so ensure that the data is always present for the function ( if you fail to pass in the correct number of arguments to a function an error will occur). Also, in some cases if you want to change the value of a variable outside the function you have to pass it to the function first.

kj2
5,933 PointsHey Andrew, thanks for the response, could you give me an example please?

Aaron Graham
18,033 Pointskj2 - A classic example of what Andrew Shook is talking about would be object getters and setters. Say you have some value in an object that you want to set. To set it, you would use an object method (a function) with some value. To get it, you would use a method with no value. Here is an example:
var myObject = {
myVal: '',
setMyVal: function (value) { this.myVal = value },
getMyVal: function() { return this.myVal }
}
To use this object, you would do the following:
var returnFromObject = '';
myObject.setMyVal("some new value");
returnFromObject = myObject.getMyVal(); //returnFromObject now contains "some new value"
It's a very contrived example, but hopefully it helps you make some sense out of this.

kj2
5,933 PointsThank you everyone for your help!
Andrew Shook
31,709 PointsAndrew Shook
31,709 PointsThanks for tagging in Jeff. I'm at work and didn't have time to respond.