Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial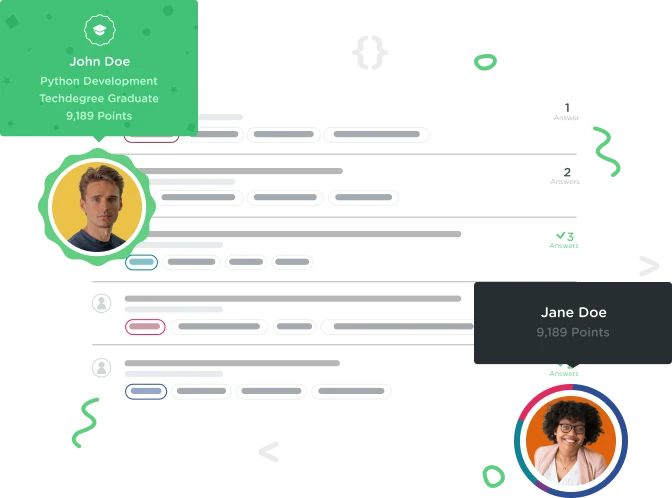

J. Ryan Conklin
22,200 PointsFunctions Return "Unable to Retrieve Results" Error
I've been following along with the videos and the Functions full_catalog_array() and random_catalog_array() do not seem to work as written. I've added the "SELECT Media.media_id" solution I found in a couple other videos to no avail. I've also tried resetting the functions file to what's available in the downloads section (these actually break more than they fix). Currently the random 4 listings return the "unable to retrieve" error as well as clicking on an item to view details. Here's what I currently have for the functions.php file:
<?php
function full_catalog_array() {
include("connection.php");
try {
$results = $db->query(
"SELECT Media.media_id, title, category, img
FROM Media");
} catch (Exception $e){
echo "Unable to retrieve results";
exit;
}
$catalog = $results->fetchAll(PDO::FETCH_ASSOC);
return $catalog;
}
function random_catalog_array() {
include("connection.php");
try {
$results = $db->query(
"SELECT Media.media_id, title, category, img
FROM Media
ORDER BY RANDOM()
LIMIT 4");
} catch (Exception $e){
echo "Unable to retrieve results";
exit;
}
$catalog = $results->fetchAll(PDO::FETCH_ASSOC);
return $catalog;
}
function single_item_array($id) {
include("connection.php");
try {
$results = $db->prepare("SELECT Media.media_id, title, category, img, format, year, genre, publisher, isbn
FROM Media
JOIN Genres ON Media.genre_id = Genres.genre_id
LEFT OUTER JOIN Books ON Media.media_id = Books.media_id
WHERE Media.media_id = ?"
);
$results->bindParam(1, $id, PDO::PARAM_INT);
$results->execute();
} catch (Exception $e){
echo "Unable to retrieve results";
exit;
}
$item = $results->fetch(PDO::FETCH_ASSOC);
if (empty($item)) return $item; // Early return
try {
$results = $db->prepare("SELECT fullname, role
FROM Media_People
JOIN People ON Media.People.people_id = People.people_id
WHERE Media_People.media_id = ?"
);
$results->bindParam(1, $id, PDO::PARAM_INT);
$results->execute();
} catch (Exception $e){
echo "Unable to retrieve results";
exit;
}
while($row = $results->fetch(PDO::FETCH_ASSOC)) {
$item[$row["row"]][] = $row["fullname"];
}
return $item;
}
function get_item_html($item) {
$output = "<li><a href='details.php?id="
. $item["media_id"] . "'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
return $output;
}
function array_category($catalog, $category) {
$output = array();
foreach ($catalog as $id => $item) {
if ($category == null OR strtolower($category) == strtolower($item["category"])) {
$sort = $item["title"];
$sort = ltrim($sort, "The ");
$sort = ltrim($sort, "A ");
$sort = ltrim($sort, "An ");
$output[$id] = $sort;
}
}
asort($output);
return array_keys($output);
}
1 Answer

Zach Slagowski
15,182 PointsUse RANDOM() instead of RAND() since we're using SQLite instead of MySQL
J. Ryan Conklin
22,200 PointsJ. Ryan Conklin
22,200 PointsHa! Thanks! The inverse was true for my case - I had to change it to RAND() instead of RANDOM() since I'm using mySQL locally. Thanks again!