Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial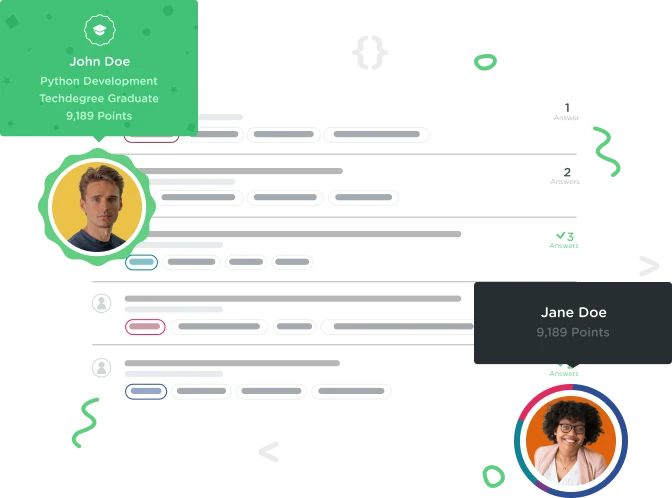

Bader Abuqamaz
2,587 PointsFunctions Vs. Methods
Hello all, I tried watching these series a couple of time. Now I now how to create a struct and how to deal with functions. However, I don't see the point of why we are creating methods from the first place if we could achieve the same thing by just creating a simple function.
1 Answer
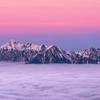
isaacrichardson
14,313 PointsHi, methods are just functions inside of an object
If you look at this example, this struct object is for counting.
struct Counter {
var count = 0
func incrementByOne() {
count += 1
}
func increment(by amount: Int) {
count += amount
}
func reset() {
count = 0
}
}
If we then assign the object to a constant
let viewCount = Counter()
We then can access , update and store properties form this object.
viewCount.count // This would display 0
viewCount.incrementByOne()
viewCount.count // This would display 1
The greatest thing about using methods and objects is that the object can be used in many different cases. We can assign this object to a new constant and update the values of count without affecting the others instances of that object
let subscribers = Counter()
viewCount.count // This would display 1 because we haven't updated the variable *count*
subscribers.count // This would display 0
subscribers.increment( amount: 10)
subscribers.count // This would display 10
In the example above us we can create a new instance of the object without changing the other instances. We can not get this functionality from using just functions.
Sorry for this being so long. Hope this helps I would play about in Xcode playground and do further tests.
Thanks
isaacrichardson
14,313 Pointsisaacrichardson
14,313 PointsHave you tried playing around in Xcode playground?