Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial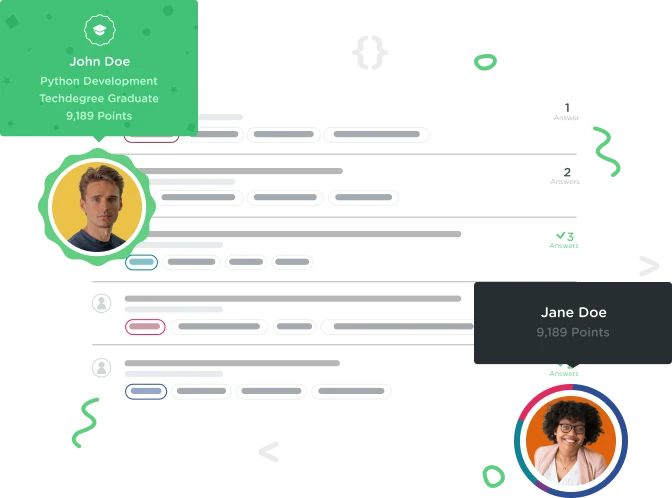
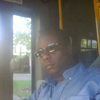
Osman Farah
1,329 PointsFunctions.py
I tried, but I can't success. So please I need help?.
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
def add_list(numbers):
for number in numbers:
return total
def summarize(add_list):
return "The sum of {} is .".format(add_list)
5 Answers

Dan Johnson
40,532 PointsFor the add_list function you have loop correct and the right idea about how to keep track of the total. Here's how to finish it up:
def add_list(numbers):
# Be mindful of the indentation, it's how Python
# recognizes blocks of code.
# Declare the total variable, intializing to 0
total = 0
for number in numbers:
# Equivalent to total = total + number
total += number
# Return outside the for block, otherwise only
# one iteration will happen.
return total
The summarize function needs to format the list and the total:
def summarize(numbers):
return "The sum of {numbers} is {total}".format(numbers=numbers, total=add_list(numbers))
format knows how to deal with lists, so you don't have to do anything extra when passing it in.
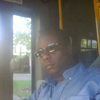
Osman Farah
1,329 PointsThanks Dan. I set up like this ,but still I can't pass. Because indent errors for return. I did tap one time and two times still won't go through. Any suggestions?.
add_list =(1, 2, 3)
def add_list(numbers):
total = 0
for number in numbers:
total += number
return total
def summarize(numbers):
return "The sum of {numbers} is {total}".format(numbers=numbers, total=add_list(numbers))

Dan Johnson
40,532 PointsAdded code tags.
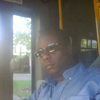
Osman Farah
1,329 PointsDan sorry return is under the total. Not what you see.
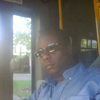
Osman Farah
1,329 PointsDan sorry return is under the total. Not what you see.

Dan Johnson
40,532 PointsIf you have total in line with the rest of the function block, then all you need to do to get it to work is move the for loop back in:
def add_list(numbers):
total = 0
for number in numbers:
total += number
return total
Also don't worry about defining your own list. The challenge will take care of that for you.
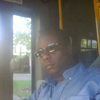
Osman Farah
1,329 PointsThanks so much Dan. Without you it will take couple days.