Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial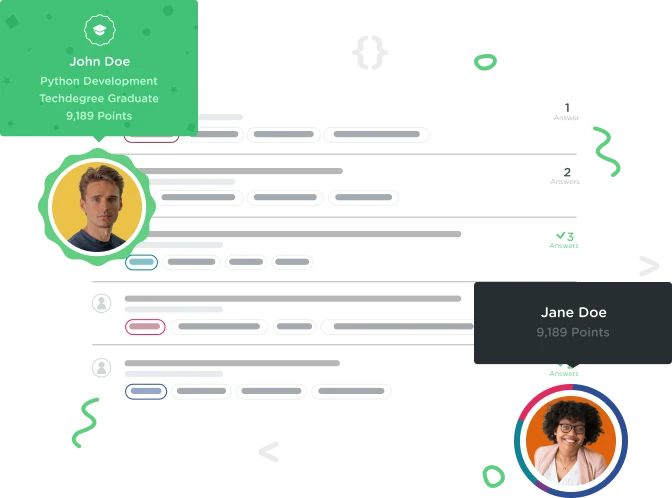

Sharad Shivmath
14,446 PointsFurther information on props.children?
Hi,
Can someone elaborate on props.children used in the video?
const GuestName = props => {
if (props.isEditing){
return (
<input type="text" value={props.children} />
);
}
return (
<span>{props.children}</span>
);
}
GuestName.PropTypes = {
isEditing: PropTypes.bool.isRequired
};
2 Answers
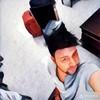
Ari Misha
19,323 PointsHiya Sharad! Lets consider an example, shall we?
<Panel title="Browse for movies">
<div>Movie stuff...</div>
<div>Movie stuff...</div>
<div>Movie stuff...</div>
<div>Movie stuff...</div>
</Panel>
You see Panel is a component in above code. This Panel has a prop for title and few divs squeezed in there as well, right? Now consider this part of code:
export default class Panel extends React.Component {
render() {
return (
<div className="panel">
<div className="panel-header">{this.props.title}</div>
<div className="panel-body">{this.props.children}</div>
</div>
);
}
}
This component is the representation of the same Panel component in ES6 syntax(duhh!). Whaddya think {this.props.children} does in above code? If you haven't guessed it yet, Then continue reading. props.children references all 'em divs children in the Panel component. Chaining children method pretty much means that you're referencing all 'em children component(s)/HTML or anything thats squeezed between Parent component(Panel component in this case). I hope it helped!
~ Ari
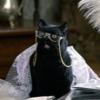
nandorkraszlan
27,542 PointsThis is the full article with these examples: https://learn.co/lessons/react-this-props-children

Chris Martinez
11,715 PointsI understand what props.children is, but i don't understand why its better in this case than just passing it as a named prop.
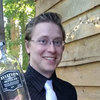
Evan Demaris
64,262 PointsHi Chris,
It sounds like you're asking why the code isn't as follows:
<GuestName isEditing={props.isEditing} value={props.name}></GuestName>
const GuestName = props => .....<span>props.value</span>
The answer is that it saves (a very small amount of) code/complexity to feed the information between tags in JSX instead. I can't see any problems that would be caused by sending it as a prop instead if you'd prefer, though.
Hope that helps!
onesoftwareengineer
Full Stack JavaScript Techdegree Graduate 19,767 Pointsonesoftwareengineer
Full Stack JavaScript Techdegree Graduate 19,767 PointsFor people who want to find out more on props.children , https://reactjs.org/docs/composition-vs-inheritance.html might be a valuable source of information.