Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial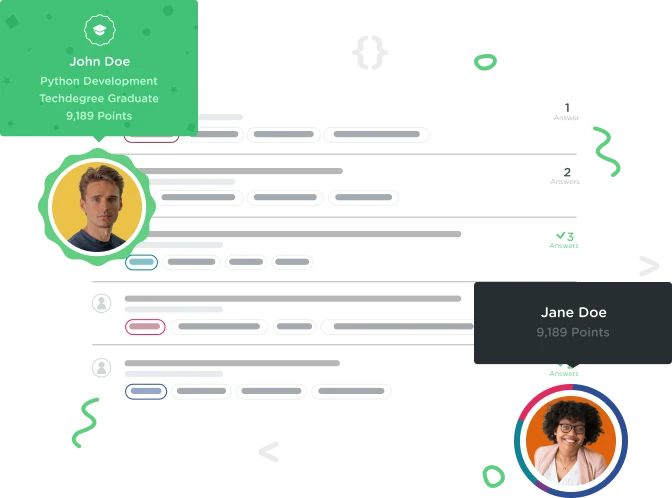

Eric Golder
2,661 PointsGame not running like it should. Get a win on first or second correct guess?
import os
import random
import sys
words =[
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def draw(bad_guesses, good_guesses, secret_word):
clear()
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
for letter in bad_guesses:
print(letter, end=' ')
print('\n\n')
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
elif not guess.isalpha():
print("You can only guess letters!")
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
print("You win!")
print("The secret word was {}".format(secret_word))
done = True
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}".format(secret_word))
done = True
if done:
play_again = input("Play again? Y/n ").lower()
if play_again != 'n':
return play(done=False)
else:
sys.exit()
def welcome():
start = input("Press enter/return to start or Q to quit ").lower()
if start == 'q':
print("Bye!")
sys.exit()
else:
return True
print('Welcome to Letter Guess!')
done = False
while True:
clear()
welcome()
play(done)
5 Answers
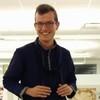
slavster
1,551 PointsEric Golder Looks like I made the same mistake as you did and after careful review of the code, it looks like we had an indentation error.
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
print("You win!")
print("The secret word was {}".format(secret_word))
done = True
Do you see how the second if is on the same indent as the first if? That should not be the case. If you re-align the second if with the For loop, it will run smoothly.
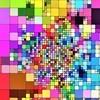
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyour while loop in the play function skips straight to the win section if you happen to guess the first letter in the word. another way might be to loop through the letters in the secret word like you're doing, and keep found = true UNTIL you hit a letter in secret word that hasn't been guessed. if that happens, found = false and play continues. if after looping through secret word, found is still true, then you have guessed the word.
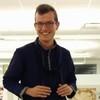
slavster
1,551 Pointsjames south My code looks the same and I'm also getting this behavior. I'm not sure I totally understand your logic, can you provide more detail?
My thinking is that the following line of code (part of the while loop in the play function) should check every single letter in the secret word against correct guesses. As long as if find one that is not, it should set found to false, right?
for letter in secret_word:
if letter not in good_guesses:
found = False
I guess I'm not entirely sure what makes the first letter unique and stops there if it finds match.
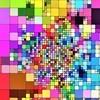
james south
Front End Web Development Techdegree Graduate 33,271 PointsEric Golder slavster my answer yesterday could have been worded better. i essentially described what he was already doing as the solution. it was a roundabout way of saying the second if statement (if found) needs to be under the for loop, not inside of it. this would accomplish what i thought i was saying - keep found true until you find an unguessed letter, if you don't find one, you have guessed the word and have won the game. lemme know if you're still stuck.

Eric Golder
2,661 PointsThanks guys

Todd Costa
3,380 PointsThank you guys, I ran into the same issue and couldn't figure it out for the life of me.