Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial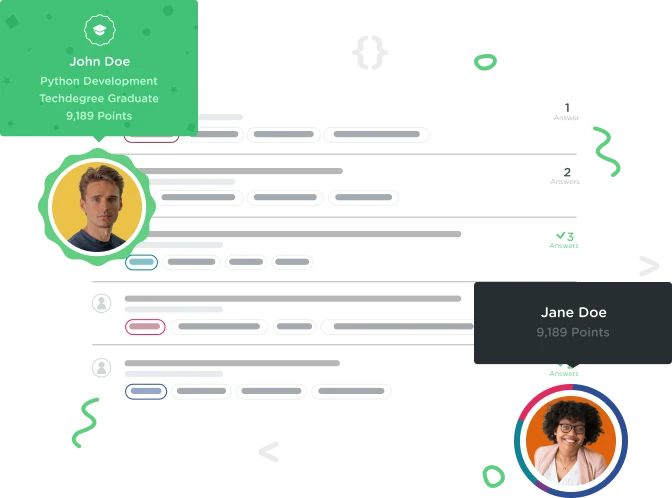

Saloni Mude
9,933 PointsGame registering first correct (good guess) letter of the word as a bad guess
Every time I try to enter the first letter of the word, it registers as a strike ie bad guess instead of adding to the word as a good guess.
For example : _ _ (Ed) It will allow me to guess the d but when I try to enter the E it will register as a strike instead of adding to the word and allowing me to win the game,
This applies to the first letter of every word. For example _ _ _ _ _ (Eddie) if i enter a "e" , it will only show up in the last letter and not in the first letter
import random
import os
import sys
#make a list of words
words = ["Rick",
"Morty",
"Steven" ,
"Aang",
"Courage",
"Korra",
"Sokka",
"Arnold",
"Mabel",
"Eddie",
"Ed" ]
def clear():
if os.name == "nt":
os.system ("cls")
else:
os.system("clear")
def draw (bad_guesses, good_guesses, secret_word):
clear()
print ("Strikes {}/7".format(len(bad_guesses)))
print("")
for letter in bad_guesses:
print(letter,end= "")
print ("\n\n")
for letter in secret_word:
if letter in good_guesses:
print(letter, end="")
else:
print("_", end ="")
print(" ")
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print ("You can only guess a single letter!")
#continue is not needed since loop will run on it's own
elif guess in bad_guesses or guess in good_guesses:
print ("You've already guessed that letter!")
#continue
elif not guess.isalpha():
print("You can only guess letters")
#continue
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found= False
if found:
print("You win!")
print("The secret word was {}".format(secret_word))
done = True
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}".format(secret_word))
done = True
if done:
play_again = input("Play again? Y/n").lower()
if play_again != "n":
return play(done = False)
else:
sys.exit()
def welcome():
start = input("Press enter to start or Q to quit").lower()
if start == "q":
print ("Bye!")
sys.exit()
else:
return True
print ("Welcome to Hangman!")
done = False
while True:
clear()
welcome()
play(done)
1 Answer
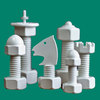
Steven Parker
231,269 Points
This game was apparently not designed to use mixed-case words.
The input is always converted to lower-case, so you can never match an upper-case letter.
I didn't try it, but I think you can adapt it work with mixed-case by changing these 3 lines:
if letter.lower() in good_guesses: # line 33
...
if guess in secret_word.lower(): # line 66
...
for letter in secret_word.lower(): # line 69
In case I missed anything, just look for other places where a conversion needs to be applied.
Saloni Mude
9,933 PointsSaloni Mude
9,933 PointsThanks a lot ! The code changes you recommended do work. The code not working because of upper-case letters didn't occur to me at all so I spent quite a while figuring out what could be wrong. Bit of an overlooked bug in the game I think.
Anyway thanks again , it was a big help :)