Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial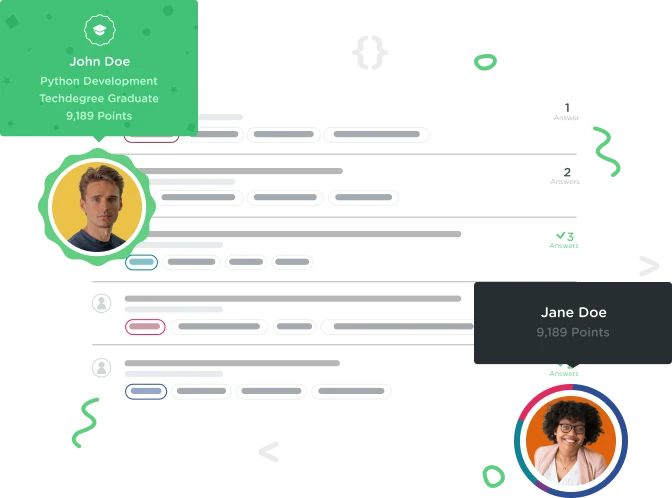

maria santiago
Courses Plus Student 142 Pointsgame run but good guesses and bad guesses does not append correctly
I triple checked and quadruple checked my codes over and over and nothing appears out of place. I ran the script in Windows PowerShell on Windows 7, Python 3.51.
Problems.
good guesses are displayed (on the line where bad guesses should be) as bad guesses and strike is counted.
bad guesses does not display, no strikes are counted
Puzzle do gets solved if good guesses matches secret word but the underscores stays untouched
import random
import os
import sys
words = [
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def draw(good_guesses, bad_guesses, secret_word):
clear()
print('Strikes: {}/7'.format(len(bad_guesses)))
print('\n\n')
for letter in bad_guesses:
print(letter, end=' ')
print('\n\n')
for letter in secret_word:
if letter in good_guesses:
print(letter, end=' ')
else:
print('_', end=' ')
print('')
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print('You can only guess a single letter:')
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter")
elif not guess.isalpha():
print('You can only guess letters only')
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
print('You win!')
print('The secret word was {}'.format(secret_word))
done = True
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}".format(secret_word))
done = True
if done:
play_again = input('Play again? Y/n '.lower())
if play_again != 'n':
return play(done=False)
else:
sys.exit()
def welcome():
start = input("Press enter/return to start or Q to quit ").lower()
if start == 'q':
print('Bye!')
sys.exit()
else:
return True
print('Welcome to Letter Guess!')
done = False
while True:
clear()
welcome()
play(done)
2 Answers
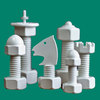
Steven Parker
230,274 PointsOn lines 62 and 78, when you call the draw function, you pass it three arguments:
draw(bad_guesses, good_guesses, secret_word)
But on line 25 where you define it, the definition shows the arguments in a different order:
def draw(good_guesses, bad_guesses, secret_word):
this causes the good guesses to be scored as bad, and vice-versa.
Also, on line 84 you have:
play_again = input('Play again? Y/n '.lower())
This causes your question to be displayed in lowercase. But you probably intended to have:
play_again = input('Play again? Y/n ').lower()
That would convert the answer to lowercase.

maria santiago
Courses Plus Student 142 PointsThanks for clearing that up in an easy to understand answer, I can finally move on with my studies. Best wishes