Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial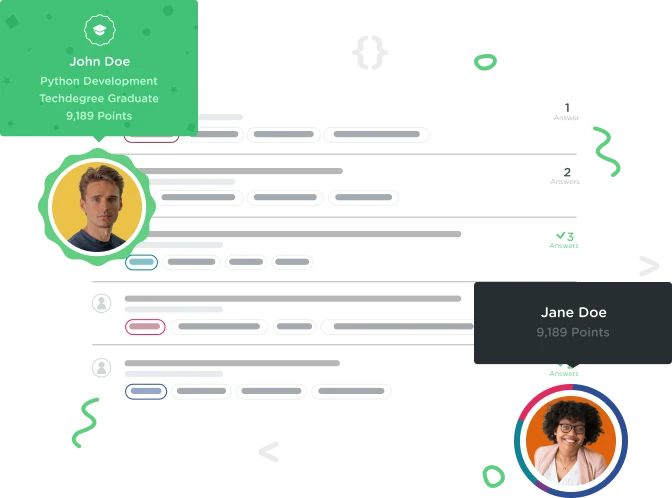
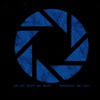
James N
17,864 Pointsgame won't trigger win or loss
i keep thinking it's something wrong with this "found" variable, but it isn't. anyways, when i play my game, it runs like normal:
Strikes: 8/7
h e q w r t u i
sony
Guess a letter:
# as you can see, the game doesn't believe i've won or lost! it just keeps on going!
Here's my workspace code:
letter_game.py
import os
import sys
import random
# Make word list
words = [
"apple",
"google",
"microsoft",
"samsung",
"nintendo",
"sony",
"sharp",
"dell",
"logitech",
"blackberry",
"bell",
"telus",
"koodo",
"rogers",
"verison",
"wind",
"netflix",
"shomi"
]
def clear():
if os.name == "nt":
os.system("cls")
else:
os.system("clear")
def draw(bad_guesses, good_guesses, secret_word):
clear()
print("Strikes: {}/7".format(len(bad_guesses)))
print("")
for letter in bad_guesses:
print(letter, end=" ")
print("\n\n")
# Draw Spaces, Draw Guessed letters, Draw Strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end="")
else:
print("_", end="")
print("")
def get_guess(bad_guesses,good_guesses):
while True:
# Take Guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess 1 letter at a time!")
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
elif not guess.isalpha():
print("You can only guess letters!")
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in good_guesses: # swapped good_guesses and secret_word, didn't help
if letter not in secret_word:
found = False
continue #deactivated found variable, didn't help
if found:
print("You win!")
print("The secret word was {}!".format(secret_word))
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}".format(secret_word))
if done:
play_again = input("Play again? Y/n ").lower()
if play_again != "n":
return play(done = False)
else:
sys.exit()
def welcome():
start = input("Press enter/return to start or Q to quit.").lower()
if start == "q":
print("Bye!")
sys.exit()
else:
return True
print("Welcome to HANGMAN!!!")
done = False
while True:
clear()
welcome()
play(done)
#while True:
# start = input("Press enter/return to start, or Q to Quit")
# if start.lower() == "q":
# break
#
# # Pick Random
# secret_word = random.choice(words)
# bad_guesses = []
# good_guesses = []
#
# while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
#
#
#
#
# if guess in secret_word:
# good_guesses.append(guess)
# if len(good_guesses) == len(list(secret_word)):
# print("You win! The word was {}".format(secret_word))
# break
#
# else:
# bad_guesses.append(guess)
# else:
# print("You didn't guess it! My secret word was {}".format(secret_word))
# # Print Win/Lose
the pile of comments at the end of the code was the old code, just in case the new one had problems.
also, there are a few comments explaining what i did to get it to work. i put everything back, though.
i had another problem with the old code, before this video. but i forgot to post it! anyways, hope you can help.
2 Answers
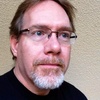
Chris Freeman
Treehouse Moderator 68,423 PointsI was able to get your code to work. The main issue was not setting done
when the word matched or strike limit was reached.
I reversed back the loops for secret_word
and good_guesses
. I also changed the continue
to a break
to stop checking once a not-guessed letter was found.
Full code:
import os
import sys
import random
# Make word list
words = [
"apple",
"google",
"microsoft",
"samsung",
"nintendo",
"sony",
"sharp",
"dell",
"logitech",
"blackberry",
"bell",
"telus",
"koodo",
"rogers",
"verison",
"wind",
"netflix",
"shomi"
]
def clear():
if os.name == "nt":
os.system("cls")
else:
os.system("clear")
def draw(bad_guesses, good_guesses, secret_word):
clear()
print("Strikes: {}/7".format(len(bad_guesses)))
print("")
for letter in bad_guesses:
print(letter, end=" ")
print("\n\n")
# Draw Spaces, Draw Guessed letters, Draw Strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end="")
else:
print("_", end="")
print("")
def get_guess(bad_guesses, good_guesses):
while True:
# Take Guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess 1 letter at a time!")
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
elif not guess.isalpha():
print("You can only guess letters!")
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word: # good_guesses: # swapped good_guesses and secret_word, didn't help
if letter not in good_guesses: # secret_word:
found = False
# continue # deactivated found variable, didn't help
break # no need to continue checking
if found:
print("You win!")
print("The secret word was {}!".format(secret_word))
done = True # Mark as done
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}".format(secret_word))
done = True # Mark as done
if done:
play_again = input("Play again? Y/n ").lower()
if play_again != "n":
return play(done=False)
else:
sys.exit()
def welcome():
start = input("Press enter/return to start or Q to quit.").lower()
if start == "q":
print("Bye!")
sys.exit()
else:
return True
print("Welcome to HANGMAN!!!")
done = False
while True:
clear()
welcome()
play(done)
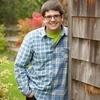
Sam Dale
6,136 PointsSee here's the interesting thing about your code. You do print out the "You lost" message. The problem is that the code clears the screen immediately and keeps going (if you are running in a terminal locally you could scroll up and see that it tells you that you lost), because you never tell the code that it's "done". All you need to do is add the line:
done = True
After the line that reads:
print("The secret word was {}".format(secret_word))
That makes your code run smoothly. Cheers!