Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial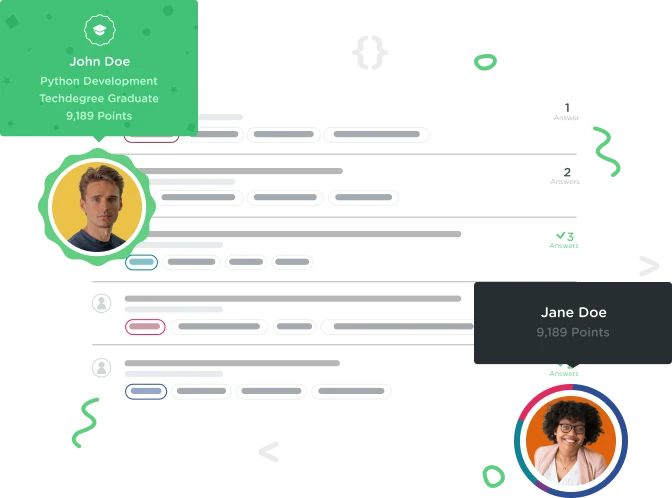

ISAIAH S
1,409 Points./Game.java:13: error: invalid method declaration; return type requir...
My error:
./Game.java:13: error: invalid method declaration; return type requir
ed
private validateGuess(char letter) {
^
./Game.java:21: error: incompatible types: unexpected return value
return letter;
^
./Game.java:25: error: cannot find symbol
letter = validateGuess(letter);
^
symbol: method validateGuess(char)
location: class Game
./Prompter.java:26: error: cannot find symbol
isHit = mGame.applyGuess(guess);
^
symbol: variable guess
location: class Prompter
4 errors
My workspaces:
Game.java:
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
private validateGuess(char letter) {
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
}
Hangman.java:
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
prompter.play();
}
}
Prompter.java:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while(mGame.getRemainingTries() > 0) {
displayProgress();
promptForGuess();
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
}
try {
isHit = mGame.applyGuess(guess);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.", iae.getMessage());
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
}
}
Thanks,
ISAIAH S
1 Answer
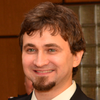
Miroslav Kovac
11,454 Pointsprivate validateGuess(char letter)
should be
private char validateGuess(char letter)

ISAIAH S
1,409 PointsThere's still an error:
./Prompter.java:26: error: cannot find symbol
isHit = mGame.applyGuess(guess);
^
symbol: variable guess
location: class Prompter
1 error
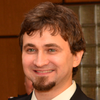
Miroslav Kovac
11,454 PointsYou have 'char guess' definition inside th while cycle. So the compiler cannot recognize the guess variable outside of cycle.
char guess; while (! isValidGuess) { String guessAsString = console.readLine("Enter a letter: "); guess = guessAsString.charAt(0); }

ISAIAH S
1,409 PointsThanks a LOT
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherProtip: If you do three back ticks and then the filename.java it does cool stuff