Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial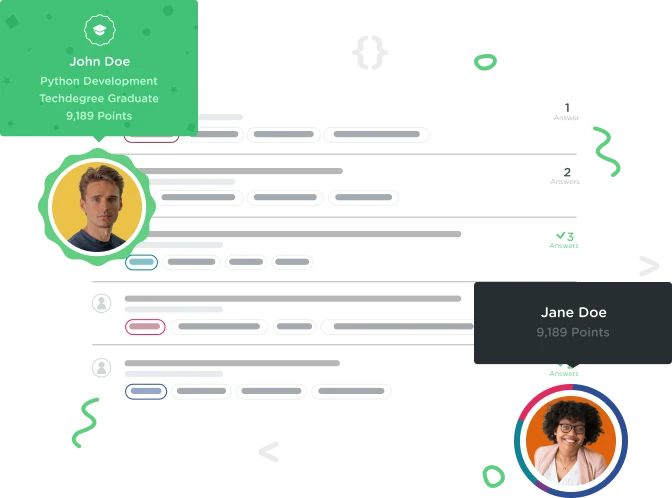

Hamzah Iqbal
Full Stack JavaScript Techdegree Student 11,145 PointsGame.js:34 Uncaught TypeError: Cannot read property 'drawHTMLToken' of undefined
class Game {
constructor() {
this.board = new Board();
this.players = this.createPlayers();
this.ready = false;
}
/**
* * Creates two player objects
* @return {Array} An array of two Player objects.
*
*
* */
createPlayers() {
const players = [new Player("Player1", 1, "#e15258", true),
new Player("Player 2", 2, "#e59a13")];
return players;
}
/* makes game ready to play
*/
startGame() {
this.board.drawHTMLBoard();
this.activePlayer.activeToken.drawHTMLToken();
this.ready = true;
}
get activePlayer() {
return this.players.find(player => player.active);
}
}
class Player {
constructor(name, id, color, active = false) {
this.name = name;
this.id = id;
this.color = color;
this.active = active;
this.token = this.createTokens(21);
}
/**
*
* @param {integer} num - number of objects to be created
*/
createTokens(num) {
const tokens = [];
for (i = 0; i < num; i++) {
let token = new Token();
tokens.push(token);
}
return tokens;
}
get unusedTokens() {
return this.tokens.filter(token => !token.dropped);
}
get activeToken() {
return this.unusedTokens[0];
}
}
class Token {
constructor(owner, index) {
this.owner = owner;
this.id = `token-$-{index}-$-{owner.id}`;
this.dropped = false;
}
drawHTMLToken() {
const div = document.createElement("div");
document.getElementById("game-board-underlay").appendChild(div);
div.setAttribute("id", this.id);
div.setAttribute("class", "token");
div.style.backgroundColor = this.owner.color;
}
}
class Board {
constructor() {
this.rows = 6;
this.columns = 7;
this.spaces = this.createSpaces();
}
createSpaces() {
const spaces = [];
for (var x = 0; x < this.rows; x++) {
const rows = [];
for (var y = 0; y < this.columns; y++) {
const space = new Space(x, y);
rows.push(space);
}
spaces.push(rows);
}
return spaces;
}
drawHTMLBoard() {
for (let column of this.spaces) {
for (let space of column) {
space.drawSVGSpace();
}
}
}
}
class Space {
constructor(x, y, diameter, radius) {
this.x = x;
this.y = y;
this.id = `space-${x}-${y}`;
this.token = null;
this.diameter = 76;
this.radius = this.diameter / 2;
}
drawSVGSpace() {
const svgSpace = document.createElementNS("http://www.w3.org/2000/svg", "circle");
svgSpace.setAttributeNS(null, "id", this.id);
svgSpace.setAttributeNS(null, "cx", (this.x * this.diameter) + this.radius);
svgSpace.setAttributeNS(null, "cy", (this.y * this.diameter) + this.radius);
svgSpace.setAttributeNS(null, "r", this.radius - 8);
svgSpace.setAttributeNS(null, "fill", "black");
svgSpace.setAttributeNS(null, "stroke", "none");
document.getElementById("mask").appendChild(svgSpace);
}
}
app.js
const newGame = new Game();
document.querySelector("#begin-game").addEventListener("click", function () {
//calls startgame on the game object initalized
newGame.startGame();
this.style.display = "none";
document.getElementById('play-area').style.opacity = '1';
});
1 Answer
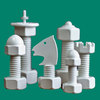
Steven Parker
231,269 PointsAs Jenner observed, the "token" (singlular) property in the Player class is being referred to as "tokens" (plural) in the "unusedTokens" method.
And for future questions, make a snapshot of your workspace and post the link to it here to facilitate easy replication of the issue (and to save a lot of space in the question!).
Jenner Thomas
8,210 PointsJenner Thomas
8,210 PointsIn your "get Unusedtokens" method, in the Player class, your return call is using the incorrect name for the 'token' property. You have 'this.token' as the property name in your constructor.